Python: Modify the attribute values of a given class
9. Create a Class Named Student with Attributes; Modify and Print Original and Modified Values
Write a Python class named Student with two attributes student_name, marks. Modify the attribute values of the said class and print the original and modified values of the said attributes.
Sample Solution:
Python Code:
class Student:
student_name = 'Terrance Morales'
marks = 93
print(f"Student Name: {getattr(Student, 'student_name')}")
print(f"Marks: {getattr(Student, 'marks')}")
setattr(Student, 'student_name', 'Angel Brooks')
setattr(Student, 'marks', 95)
print(f"Student Name: {getattr(Student, 'student_name')}")
print(f"Marks: {getattr(Student, 'marks')}")
Sample Output:
Student Name: Terrance Morales Marks: 93 Student Name: Angel Brooks Marks: 95
Flowchart:
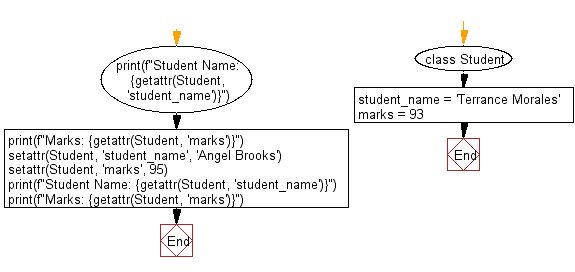
For more Practice: Solve these Related Problems:
- Write a Python class Student with attributes student_name and marks, then create an instance and modify these attributes before printing both versions.
- Write a Python program to instantiate a Student class, update its attributes using setter methods, and print before and after values.
- Write a Python program to use __dict__ to display a Student object's attribute values before and after modification.
- Write a Python program to implement a function that creates a Student instance, changes its attributes, and prints a formatted report of the changes.
Go to:
Previous: Write a Python program to crate two empty classes, Student and Marks. Now create some instances and check whether they are instances of the said classes or not. Also, check whether the said classes are subclasses of the built-in object class or not.
Next: Write a Python class named Student with two attributes student_id, student_name. Add a new attribute student_class and display the entire attribute and their values of the said class. Now remove the student_name attribute and display the entire attribute with values.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.