Python: Count the number of students of individual class
33. Count the Number of Students in an Individual Class
Write a Python program to count the number of students in an individual class.
Sample Solution:
Python Code:
# Import the Counter class from the collections module
from collections import Counter
# Create a tuple 'classes' containing class names and the number of students in each class
classes = (
('V', 1),
('VI', 1),
('V', 2),
('VI', 2),
('VI', 3),
('VII', 1),
)
# Create a Counter object 'students' to count the occurrences of class names in 'classes'
students = Counter(class_name for class_name, no_students in classes)
# Print the 'students' Counter object, which represents the count of students in each class
print(students)
Sample Output:
Counter({'VI': 3, 'V': 2, 'VII': 1})
Flowchart:
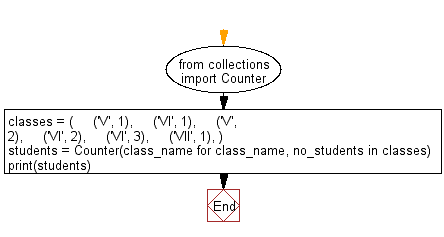
For more Practice: Solve these Related Problems:
- Write a Python program to use collections.Counter to count the frequency of class names from a list of student records.
- Write a Python program to iterate over a dictionary of student classes and output the count of students per class.
- Write a Python program to implement a function that takes a list of class identifiers and returns the count of students in each class.
- Write a Python program to use dictionary comprehension to build a frequency dictionary for classes from a tuple-of-tuples.
Go to:
Previous: Write a Python program to find the class wise roll number from a tuple-of-tuples.
Next: Write a Python program to create an instance of an OrderedDict using a given dictionary. Sort the dictionary during the creation and print the members of the dictionary in reverse order.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.