Python Cyber Security - Program for a Brute-Force Attack on Passwords
10. Brute-Force Attack Simulator
In cryptography, a brute-force attack consists of an attacker submitting many passwords or passphrases with the hope of eventually guessing correctly. The attacker systematically checks all possible passwords and passphrases until the correct one is found. Alternatively, the attacker can attempt to guess the key which is typically created from the password using a key derivation function. This is known as an exhaustive key search.
Write a Python program that simulates a brute-force attack on a password by trying out all possible character combinations.
Note: The following method of cracking passwords can be very time-consuming, especially for longer passwords or if the set of possible characters is large. In practice, attackers often use more sophisticated methods such as dictionary attacks or rainbow table attacks. It is highly recommended to use strong passwords to protect sensitive information.
Sample Solution:
Python Code:
import itertools
import string
def bruteforce_attack(password):
chars = string.printable.strip()
attempts = 0
for length in range(1, len(password) + 1):
for guess in itertools.product(chars, repeat=length):
attempts += 1
guess = ''.join(guess)
if guess == password:
return (attempts, guess)
return (attempts, None)
password = input("Input the password to crack: ")
attempts, guess = bruteforce_attack(password)
if guess:
print(f"Password cracked in {attempts} attempts. The password is {guess}.")
else:
print(f"Password not cracked after {attempts} attempts.")
Sample Output:
Input the password to crack: pass Password cracked in 21695135 attempts. The password is pass. Input the password to crack: ade# Password cracked in 9261603 attempts. The password is ade#.
Explanation:
In the above exercise we utilize the string module to get all possible characters that could be used in a password. The itertools.product function generates all possible combinations of characters up to the password length. The program then checks each combination to see if it matches the password. The number of attempts to crack the password is recorded and printed out at the end of the program
Flowchart:
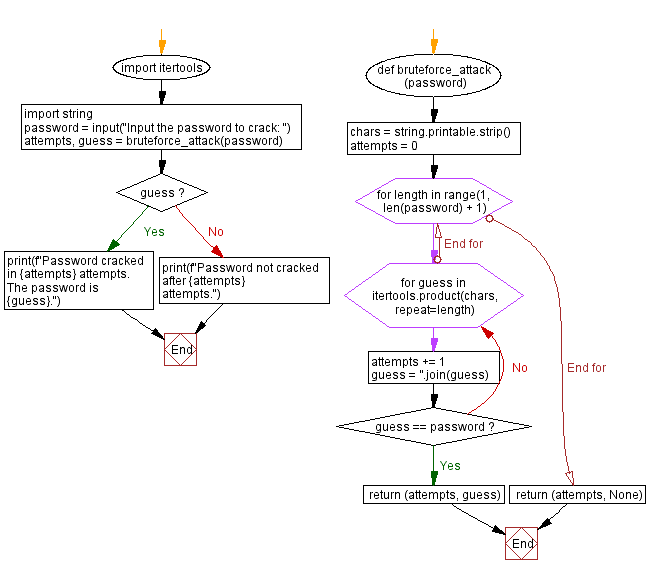
For more Practice: Solve these Related Problems:
- Write a Python program to simulate a brute-force password attack by generating all possible combinations of characters up to a certain length and checking each against a target hash.
- Write a Python function that recursively generates all possible strings of a given length from a set of characters and compares them to a known password.
- Write a Python script to measure the time taken to brute-force a password of a specified length and output the elapsed time and number of attempts.
- Write a Python program to simulate a brute-force attack with multithreading to try different character combinations concurrently, and then print the result when the correct password is found.
Go to:
Previous: Program for simulating dictionary attack on password.
Next: Python Generator and Yiels Exercises Home.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.