Python: Get current time in milliseconds
12. Time in Milliseconds
Write a Python program to get the current time in milliseconds in Python.
Sample Solution:
Python Code:
# Import the time module
import time
# Get the current time in seconds since the epoch using time.time(),
# then multiply it by 1000 to convert seconds to milliseconds,
# round the result to the nearest integer, and store it in the variable 'milli_sec'
milli_sec = int(round(time.time() * 1000))
# Print the current time in milliseconds
print(milli_sec)
Output:
1494055960573
Explanation:
In the exercise above,
- The code imports the "time" module.
- Calculating milliseconds:
- It retrieves the current time in seconds since the epoch (January 1, 1970) using "time.time()".
- The current time in seconds is multiplied by 1000 to convert it to milliseconds, as there are 1000 milliseconds in a second.
- The result is rounded to the nearest integer using the "round()" function to ensure a whole number.
- The rounded value representing the current time in milliseconds is stored in the variable 'milli_sec'.
- Finally it prints the value stored in 'milli_sec', which represents the current time in milliseconds since the epoch.
Flowchart:
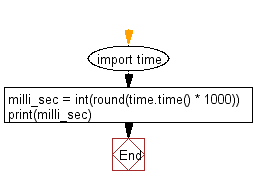
For more Practice: Solve these Related Problems:
- Write a Python program to fetch the current time and then display it in milliseconds since the epoch.
- Write a Python script to get the current time in milliseconds and then compare it with a given timestamp to compute the elapsed time.
- Write a Python program that converts the current time to milliseconds, then formats it into a human-readable string.
- Write a Python function to display the current local time in milliseconds and then compute the difference from the current UTC time in milliseconds.
Go to:
Previous: Write a Python program to convert Year/Month/Day to Day of Year.
Next: Write a Python program to get week number.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.