Python: Get days between two dates
18. Days Between Dates
Write a Python program to get days between two dates.
Sample Solution:
Python Code:
# Import the date class from the datetime module
from datetime import date
# Create a date object representing February 28, 2000 and assign it to variable 'a'
a = date(2000, 2, 28)
# Create a date object representing February 28, 2001 and assign it to variable 'b'
b = date(2001, 2, 28)
# Print the difference between the dates 'b' and 'a'
print(b - a)
Output:
366 days, 0:00:00
Explanation:
In the exercise above,
- The code imports the "date" class from the "datetime" module.
- Creating date objects:
- It creates two date objects:
- 'a' representing February 28, 2000.
- 'b' representing February 28, 2001.
- Calculating the date difference:
- It calculates the difference between the two dates (b - a), which results in a 'timedelta' object representing the duration between the two dates
- Finally, it prints the difference between the two dates, which is the duration between February 28, 2000, and February 28, 2001.
Flowchart:
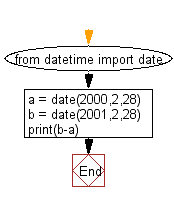
For more Practice: Solve these Related Problems:
- Write a Python program to calculate the number of days between two input dates and then output the result in days and weeks.
- Write a Python script that takes two dates, computes the timedelta between them, and then displays the total number of days along with hours.
- Write a Python function to compute and print the difference in days between two dates, also handling cases where the first date is later than the second.
- Write a Python program to calculate the days between two dates and then determine if that difference is a prime number.
Go to:
Previous: Write a Python program to drop microseconds from datetime.
Next: Write a Python program to get the date of the last Tuesday.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.