Python: Add a month with a specified date
23. Add Month to Date
Write a Python program to add a month to a specified date.
Sample Solution:
Python Code:
# Import the date and timedelta classes from the datetime module
from datetime import date, timedelta
# Import the calendar module
import calendar
# Define the start date as December 25, 2014
start_date = date(2014, 12, 25)
# Get the number of days in the month of the start date's year and month
# The monthrange() function returns a tuple containing the weekday of the first day of the month
# and the number of days in the month, and we access the second value ([1]) which represents the number of days
days_in_month = calendar.monthrange(start_date.year, start_date.month)[1]
# Print the date obtained by adding the number of days in the month to the start date
print(start_date + timedelta(days=days_in_month))
Output:
2015-01-25
Explanation:
In the exercise above,
- The code imports the "date" and "timedelta" classes from the "datetime" module. It also imports the "calendar" module.
- Defined start date:
- It defines a variable 'start_date' with the value representing December 25, 2014.
- Calculating days in the month:
- It uses the "monthrange()" function from the "calendar" module to determine the number of days in the month of the start date's year and month.
- The "monthrange()" function returns a tuple containing the weekday of the first day of the month and the number of days in the month. By accessing the second value of this tuple, it retrieves the number of days in the month.
- Calculating the end date:
- It calculates the end date by adding the number of days in the month to the start date using timedelta(days=days_in_month).
- It prints the calculated end date.
Flowchart:
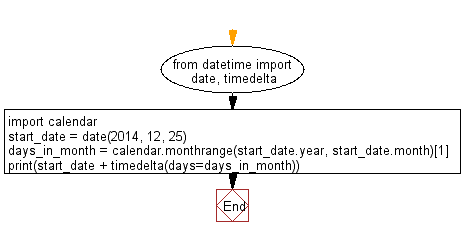
For more Practice: Solve these Related Problems:
- Write a Python program to add one calendar month to a given date, correctly handling year transitions.
- Write a Python function to add a specified number of months to a date and then format the new date as "YYYY-MM-DD".
- Write a Python script to compute the date one month later and then adjust for shorter months (e.g., Jan 31 becomes Feb 28/29).
- Write a Python program to add a month to a date and then check if the new date falls on the same day of the week as the original.
Go to:
Previous: Write a Python program to get the number of days of a given month and year.
Next: Write a Python program to count the number of Monday of the 1st day of the month from 2015 to 2016.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.