Python: Calculate a number of days between two dates
32. Days Between Two Dates
Write a Python program to calculate the number of days between two dates.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Import the date class from datetime module
from datetime import date
# Define a function called differ_days which calculates the difference in days between two dates
def differ_days(date1, date2):
# Assign date1 to variable a
a = date1
# Assign date2 to variable b
b = date2
# Return the difference in days between the two dates
return (a - b).days
# Print an empty line
print()
# Print the difference in days between October 12, 2016 and December 10, 2015
print(differ_days((date(2016, 10, 12)), date(2015, 12, 10)))
# Print the difference in days between March 23, 2016 and December 10, 2017
print(differ_days((date(2016, 3, 23)), date(2017, 12, 10)))
# Print an empty line
print()
Output:
307 -627
Explanation:
In the exercise above,
- The code imports the "datetime" module.
- It defines a function named "differ_days()" that takes two date objects (date1 and date2) as input and returns the difference in days between them.
- Inside the function:
- It assigns the input dates 'date1' and 'date2' to variables 'a' and 'b' respectively.
- It calculates the difference in days between the two dates using the 'days' attribute of the "timedelta" object obtained by subtracting 'b' from 'a'.
- It returns the difference in days.
- Then, it calls the "differ_days()" function twice:
- First, it calculates and prints the difference in days between October 12, 2016, and December 10, 2015.
- Second, it calculates and prints the difference in days between March 23, 2016, and December 10, 2017.
Flowchart:
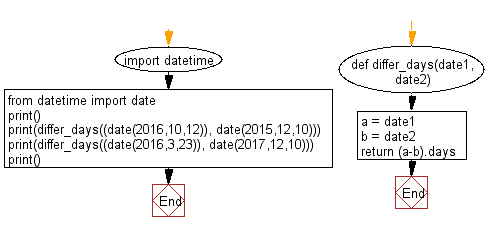
For more Practice: Solve these Related Problems:
- Write a Python program to calculate the exact number of days between two dates provided in "YYYY-MM-DD" format.
- Write a Python script to compute the day difference between any two user-input dates and then display the result in days.
- Write a Python function to find the number of days between two dates and then determine if that number is even or odd.
- Write a Python program to calculate and print the number of days between two dates, along with the number of full weeks contained within.
Go to:
Previous: Write a Python program convert a string date to the timestamp.
Next: Write a Python program to calculate number of days between two datetimes.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.