Python: Convert a given time in seconds since the epoch to a string representing local time
59. Epoch Seconds to Local Time
Write a Python program to convert a given time in seconds since the epoch to a string representing local time.
Sample Solution:
Python Code:
# Import the time module
import time
# Print the current time in a human-readable format using ctime()
print(time.ctime())
# Print the time corresponding to the given timestamp (236543789) in a human-readable format using ctime()
print(time.ctime(236543789))
Output:
Tue Apr 13 11:51:51 2021 Thu Jun 30 18:36:29 1977
Explanation:
In the exercise above,
- The code imports the "time" module.
- It prints the current time in a human-readable format using the ctime() function without arguments. This function returns a string representing the current local time in the format 'Day Month Date HH:MM:SS Year'.
- It prints the time corresponding to the given timestamp (236543789) in a human-readable format using the "ctime()" function with the timestamp as an argument. This function returns a string representing the local time corresponding to the given timestamp in the same format as mentioned above.
Flowchart:
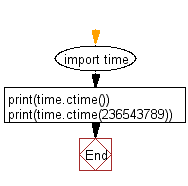
For more Practice: Solve these Related Problems:
- Write a Python program to convert a given number of seconds since the epoch to a local time string using time.ctime.
- Write a Python script to take an input of epoch seconds and output the corresponding local date and time in "YYYY-MM-DD HH:MM:SS" format.
- Write a Python function to convert seconds since the epoch to a local datetime and then print the day of the week.
- Write a Python program to read a Unix timestamp from the user, convert it to local time, and then compare it with the current local time.
Go to:
Previous: Write a Python program that can suspend execution of a given script a given number of seconds.
Next: Write a Python program to print simple format of time, full names and the representation format and preferred date time format.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.