Python: Print simple format of time, full names and the representation format and preferred date time format
Python Datetime: Exercise-60 with Solution
Write a Python program that prints the time, names, representation format, and the preferred date time format in a simple format.
Sample Solution:
Python Code:
# Import the time module
import time
# Print a message indicating simple format of time
print("\nSimple format of time:")
# Format the current time in a specific format and store it in variable s
s = time.strftime("%a, %d %b %Y %H:%M:%S + 1010", time.gmtime())
# Print the formatted time
print(s)
# Print a message indicating full names and the representation
print("\nFull names and the representation:")
# Format the current time in a specific format and store it in variable s
s = time.strftime("%A, %D %B %Y %H:%M:%S + 0000", time.gmtime())
# Print the formatted time
print(s)
# Print a message indicating preferred date time format
print("\nPreferred date time format:")
# Format the current time in the preferred format and store it in variable s
s = time.strftime("%c")
# Print the formatted time
print(s)
# Format the current time to include date, time, year, and year in variable s
s = time.strftime("%x, %X, %y, %Y")
# Print an example message followed by the formatted time
print("Example 11:", s)
Output:
Simple format of time: Tue, 13 Apr 2021 12:02:01 + 1010 Full names and the representation: Tuesday, 04/13/21 April 2021 12:02:01 + 0000 Preferred date time format: Tue Apr 13 12:02:01 2021 Example 11: 04/13/21, 12:02:01, 21, 2021
Explanation:
In the exercise above,
- The code imports the "time" module.
- It prints a message indicating the simple format of time.
- It formats the current time in a specific format using "time.strftime()" with %a, %d %b %Y %H:%M:%S + 1010 format and stores it in the variable 's'.
- It prints the formatted time stored in the variable 's'.
- It prints a message indicating full names and the representation.
- It formats the current time in another specific format using "time.strftime()" with %A, %D %B %Y %H:%M:%S + 0000 format and stores it in the variable 's'.
- It prints the formatted time stored in the variable 's'.
- It prints a message indicating the preferred date time format.
- It formats the current time in the preferred format (%c) and stores it in the variable 's'.
- It prints the formatted time stored in the variable 's'.
- It formats the current time to include date, time, year, and year in the variable 's'.
- It prints an example message followed by the formatted time stored in the variable 's'.
Flowchart:
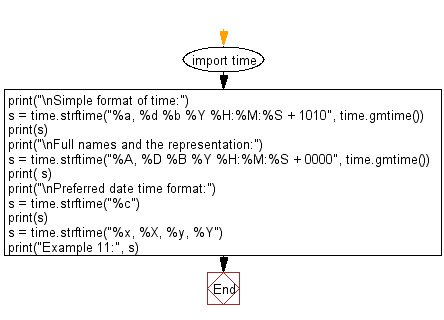
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to convert a given time in seconds since the epoch to a string representing local time.
Next: Write a Python program that takes a given number of seconds and pass since epoch as an argument. Print structure time in local time.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/date-time-exercise/python-date-time-exercise-60.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics