Python Function: Convert bytearray to bytes
Python Bytes and Byte Arrays Data Type: Exercise-10 with Solution
Write a Python function that converts a bytearray to a bytes object.
Sample Solution:
Code:
def bytearray_to_bytes(bytearray_obj):
bytes_obj = bytes(bytearray_obj)
return bytes_obj
def main():
try:
original_bytearray = bytearray([12, 51, 10, 15, 95, 102, 132, 117])
bytes_result = bytearray_to_bytes(original_bytearray)
print("Original Bytearray:", original_bytearray)
print("Bytes Object:", bytes_result)
print("Decoded String:", bytes_result.decode("utf-8"))
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Original Bytearray: bytearray(b'\x0c3\n\x0f_f\x84u') Bytes Object: b'\x0c3\n\x0f_f\x84u' An * error occurred: 'utf-8' codec can't decode byte 0x84 in position 6: invalid start byte
In the above exercise, the "bytearray_to_bytes()" function takes a bytearray as input and converts it into a bytes object using the bytes() constructor. In the "main()" function, a sample bytearray is provided, converted to a bytes object, and the decoded string is printed.
Flowchart:
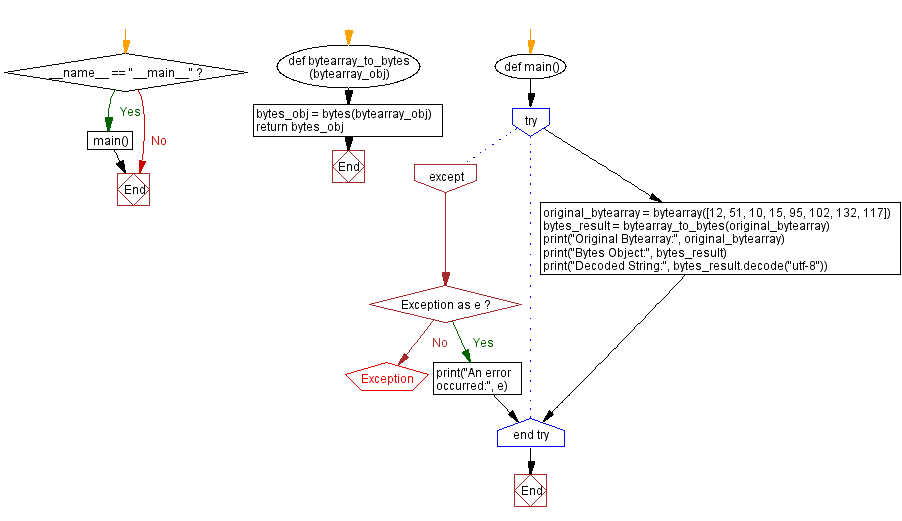
Previous: Search bytes sequence in file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics