Python random number generator using generators
Python: Generators Yield Exercise-2 with Solution
Write a Python program to implement a generator that generates random numbers within a given range.
Sample Solution:
Python Code:
import random
def random_number_generator(start, end):
while True:
yield random.randint(start, end)
# Accept input from the user
start = int(input("Input the start value: "))
end = int(input("Input the end value: "))
# Create the generator object
random_numbers = random_number_generator(start, end)
# Generate and print 10 random numbers
print("Random numbers between",start,"and",end)
for _ in range(10):
print(next(random_numbers))
Sample Output:
Input the start value: 1 Input the end value: 10 Random numbers between 1 and 10 10 6 4 6 6 5 6 2 8 9
Input the start value: 445 Input the end value: 760 Random numbers between 445 and 760 489 687 611 695 731 455 504 567 704 468
Explanation:
In the above exercise,
- At first the code imports the random module, which provides functions for generating random numbers.
- The "random_number_generator(start, end)" function is defined as a generator. It uses a while loop to continuously generate random numbers within the given range. It uses the yield keyword to generate random numbers.
- The program prompts the user to input the start and end values for the random numbers range.
- The generator object random_numbers is created by calling the random_number_generator function with the start and end values.
- The program then iterates over the generator using a for loop. Here, it generates and prints 10 random numbers by calling next(random_numbers).
Flowchart:
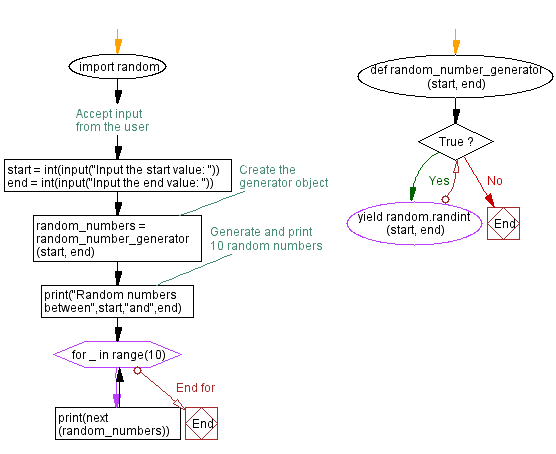
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Yielding cubes from 1 to n.
Next: Python prime number generator using generators.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics