Python prime number generator using generators
Python: Generators Yield Exercise-3 with Solution
Write a Python program that creates a generator function that generates all prime numbers between two given numbers.
A prime number (or a prime) is a natural number greater than 1 that is not a product of two smaller natural numbers. A natural number greater than 1 that is not prime is called a composite number. For example, 5 is prime because the only ways of writing it as a product, 1 × 5 or 5 × 1.
Sample Solution:
Python Code:
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
def prime_nums_generator():
n = 2
while True:
if is_prime(n):
yield n
n += 1
# Create the generator object
primes = prime_nums_generator()
# Accept input from the user
n = int(input("Input the number of prime numbers you want to generate? "))
# Generate and print the first 10 prime numbers
print("First",n,"Prime numbers:")
for _ in range(n):
print(next(primes))
Sample Output:
Input the number of prime numbers you want to generate? 12 First 12 Prime numbers: 2 3 5 7 11 13 17 19 23 29 31 37
Explanation:
In the above exercise,
- The is_prime(n) function checks whether a number n is prime.
- The prime_nums_generator() function is a generator. It initializes n to 2, the first prime number, and enters an infinite loop. It checks if n is prime using the is_prime() function, and if so, it yields n. Then, it increments n by 1 and continues the loop.
- The generator object primes is created by calling the prime_nums_generator() function.
- The program prompts the user to input the number of prime numbers they wish to generate and stores it in the variable n.
- The program then iterates over the generator using a for loop. In this case, it generates and prints the specified number of prime numbers by calling next(primes).
Flowchart:
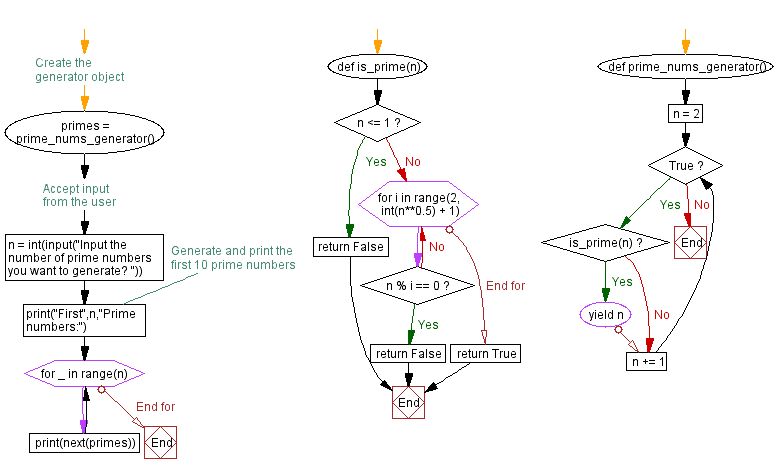
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Python random number generator using generators.
Next: Python Fibonacci generator using generators.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics