Python Fibonacci generator using generators
Python: Generators Yield Exercise-4 with Solution
Write a Python program to implement a generator function that generates the Fibonacci sequence.
In mathematics, the Fibonacci sequence is a sequence in which each number is the sum of the two preceding ones. Numbers that are part of the Fibonacci sequence are known as Fibonacci numbers. Starting from 0 and 1, the first few values in the sequence are: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144.
Sample Solution:
Python Code:
def fibonacci():
x, y = 0, 1
while True:
yield x
x, y = y, x + y
# Accept input from the user
n = int(input("Input the number of Fibonacci numbers you want to generate? "))
print("Number of first ",n,"Fibonacci numbers:")
fib = fibonacci()
for _ in range(n):
print(next(fib),end=" ")
Sample Output:
Input the number of Fibonacci numbers you want to generate? 4 Number of first 4 Fibonacci numbers: 0 1 1 2
Input the number of Fibonacci numbers you want to generate? 10 Number of first 10 Fibonacci numbers: 0 1 1 2 3 5 8 13 21 34
Explanation:
In the above exercise,
- The fibonacci() function is defined as a generator function. It initializes two variables x and y with 0 and 1, respectively.
- The function enters an infinite loop using while True.
- Inside the loop, it uses the yield keyword to yield the current value of x. This represents the Fibonacci number in the sequence.
- After yielding a, the function updates x and y by swapping their values: x becomes y, and y becomes the sum of the previous values of x and y.
- The loop continues, generating the next Fibonacci number each time yield is encountered.
- To use this generator and print the Fibonacci sequence, iterate over it using a loop. Use the next() function to manually retrieve the next Fibonacci number.
Flowchart:
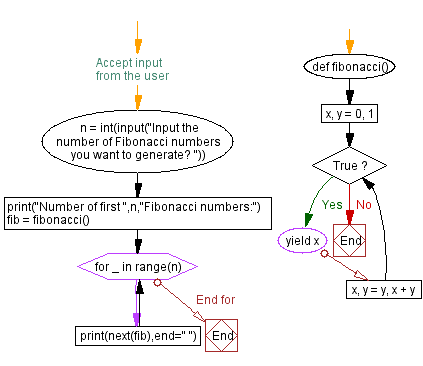
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Python prime number generator using generators.
Next: Python permutations generator using generators.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/generators-yield/python-generators-yield-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics