Python: Combine two given sorted lists using heapq module
20. Merge Two Sorted Lists
Write a Python program to combine two sorted lists using the heapq module.
Sample Solution:
Python Code:
from heapq import merge
nums1 = [1, 3, 5, 7, 9, 11]
nums2 = [0, 2, 4, 6, 8, 10]
print("Original sorted lists:")
print(nums1)
print(nums2)
print("\nAfter merging the said two sorted lists:")
print(list(merge(nums1, nums2)))
Sample Output:
Original sorted lists: [1, 3, 5, 7, 9, 11] [0, 2, 4, 6, 8, 10] After merging the said two sorted lists: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
Flowchart:
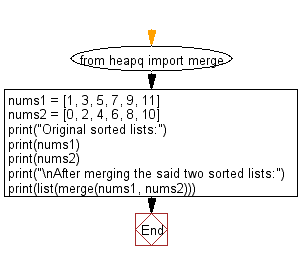
For more Practice: Solve these Related Problems:
- Write a Python program to merge two sorted lists into one sorted list using heapq.merge and then print the merged result.
- Write a Python script to combine two sorted arrays using heapq.merge and verify the sorted order of the output.
- Write a Python function to merge two sorted lists with heapq and then compare the result with the manual merge algorithm.
- Write a Python program to use heapq.merge to merge two sorted lists and then output the merged list in a single line.
Go to:
Previous: Write a Python program to print a heap as a tree-like data structure.
Next: Write a Python program to push three items into the heap and print the items from the heap.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.