Python: Push three items into the heap and print the items from the heap
21. Push Three Items to Heap
Write a Python program to push three items into the heap and print the items from the heap.
Sample Solution:
Python Code:
import heapq
heap = []
heapq.heappush(heap, ('V', 1))
heapq.heappush(heap, ('V', 2))
heapq.heappush(heap, ('V', 3))
for a in heap:
print(a)
Sample Output:
('V', 1) ('V', 2) ('V', 3)
Flowchart:
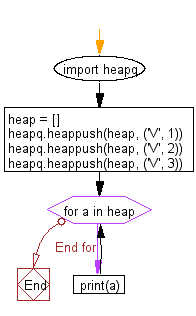
For more Practice: Solve these Related Problems:
- Write a Python program to push three items onto a heap using heapq.heappush and then iterate over the heap to print each item.
- Write a Python script to insert three specific elements into a heap and then print the heap list to show its internal structure.
- Write a Python program to add three tuples into a heap and then use heapq.nsmallest to output all the items in order.
- Write a Python function to push three items into a heap and then print the heap to verify that the smallest element is at the root.
Go to:
Previous: Write a Python program to combine two given sorted lists using heapq module.
Next: Write a Python program to push three items into a heap and return the smallest item from the heap. Also Pop and return the smallest item from the heap.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.