Python: Push three items into a heap and return the smallest item from the heap
22. Push & Pop Smallest
Write a Python program to push three items into a heap and return the smallest item from the heap. Also, pop and return the smallest item from the heap.
Sample Solution:
Python Code:
import heapq
heap = []
heapq.heappush(heap, ('V', 3))
heapq.heappush(heap, ('V', 2))
heapq.heappush(heap, ('V', 1))
print("Items in the heap:")
for a in heap:
print(a)
print("----------------------")
print("The smallest item in the heap:")
print(heap[0])
print("----------------------")
print("Pop the smallest item in the heap:")
heapq.heappop(heap)
for a in heap:
print(a)
Sample Output:
Items in the heap: ('V', 1) ('V', 3) ('V', 2) ---------------------- The smallest item in the heap: ('V', 1) ---------------------- Pop the smallest item in the heap: ('V', 2) ('V', 3)
Flowchart:
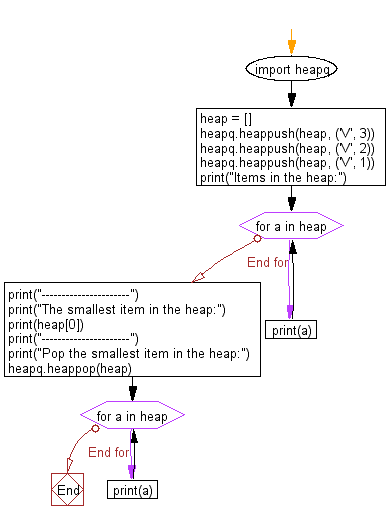
For more Practice: Solve these Related Problems:
- Write a Python program to push three items into a heap, then retrieve and print the smallest item using heapq.heappushpop.
- Write a Python script to add three items to a heap, print the heap, then pop the smallest element and display the updated heap.
- Write a Python program to insert three elements into a heap, output the smallest item without removal, and then remove it with heappop.
- Write a Python function to simulate a heap update by pushing items, returning the smallest element, and printing the final heap structure.
Go to:
Previous: Write a Python program to push three items into the heap and print the items from the heap.
Next: Write a Python program to push an item on the heap, then pop and return the smallest item from the heap.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.