Python: Push an item on the heap, then pop and return the smallest item from the heap
23. Heappushpop Operation
Write a Python program to push an item on the heap, then pop and return the smallest item from the heap.
Sample Solution:
Python Code:
import heapq
heap = []
heapq.heappush(heap, ('V', 3))
heapq.heappush(heap, ('V', 2))
heapq.heappush(heap, ('V', 1))
print("Items in the heap:")
for a in heap:
print(a)
print("----------------------")
print("Using heappushpop push item on the heap and return the smallest item.")
heapq.heappushpop(heap, ('V', 6))
for a in heap:
print(a)
Sample Output:
Items in the heap: ('V', 1) ('V', 3) ('V', 2) ---------------------- Using heappushpop push item on the heap and return the smallest item. ('V', 2) ('V', 3) ('V', 6)
Flowchart:
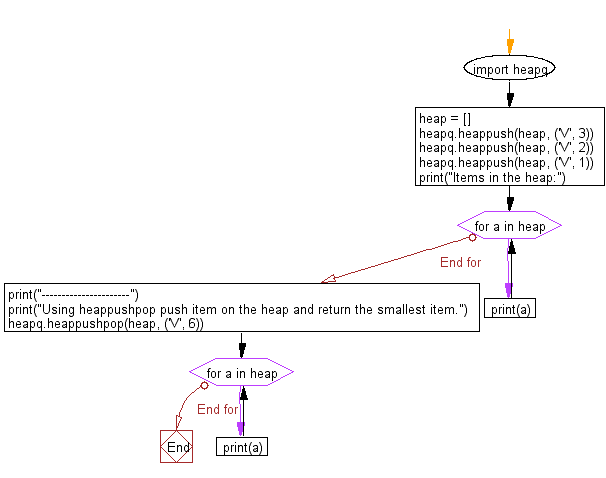
For more Practice: Solve these Related Problems:
- Write a Python program to push an item onto a heap and then immediately pop the smallest item using heapq.heappushpop, printing both results.
- Write a Python script to demonstrate the heappushpop function by inserting an element and then returning the minimum element, showing the heap before and after.
- Write a Python program to add an item to an existing heap using heappushpop and then print the smallest element returned and the modified heap.
- Write a Python function to perform heappushpop on a heap and then verify the returned value is indeed the smallest among the original items.
Go to:
Previous: Write a Python program to push three items into a heap and return the smallest item from the heap. Also Pop and return the smallest item from the heap.
Next: Write a Python program to create a heapsort, pushing all values onto a heap and then popping off the smallest values one at a time.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.