Python: Create a LIFO queue
29. LIFO Queue (Stack) Creation
Write a Python program to create a LIFO queue.
Sample Solution:
Python Code:
import queue
q = queue.LifoQueue()
#insert items at the head of the queue
for x in range(4):
q.put(str(x))
#remove items from the head of the queue
while not q.empty():
print(q.get(), end=" ")
print()
Sample Output:
3 2 1 0
Flowchart:
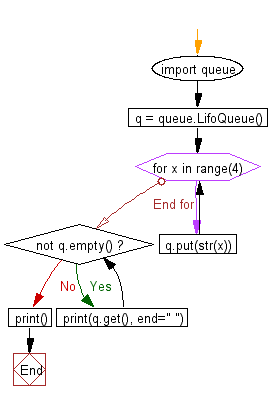
For more Practice: Solve these Related Problems:
- Write a Python program to create a LIFO queue (stack) using a list, push several elements, and then pop them in reverse order, printing each element. .
- Write a Python script to simulate a stack by appending items to a list and then removing them using pop(), outputting the items in LIFO order.
- Write a Python program to implement a LIFO queue and demonstrate its behavior by printing the state of the stack before and after each pop operation.
- Write a Python function to create a LIFO queue, push a series of elements, and then return the list of elements in the order they are popped.
Go to:
Previous: Write a Python program to create a FIFO queue.
Next: Python Bisect Exercises Home.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.