Python: Pushing all values onto a heap and then popping off the smallest values one at a time
Python heap queue algorithm: Exercise-3 with Solution
Write a Python program to implement heapsort by pushing all values onto a heap and then popping off the smallest values one at a time.
Sample Solution:
Python Code:
import heapq as hq
def heapsort(iterable):
h = []
for value in iterable:
hq.heappush(h, value)
return [hq.heappop(h) for i in range(len(h))]
print(heapsort([1, 3, 5, 7, 9, 2, 4, 6, 8, 0]))
Sample Output:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Flowchart:
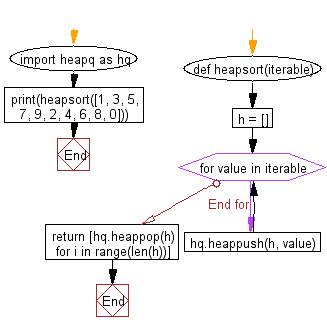
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the three smallest integers from a given list of numbers using Heap queue algorithm.
Next: Write a Python function which accepts an arbitrary list and converts it to a heap using Heap queue algorithm.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics