Python: Accepts an arbitrary list and converts it to a heap using Heap queue algorithm
Python heap queue algorithm: Exercise-4 with Solution
Write a Python function that accepts an arbitrary list and converts it to a heap using the heap queue algorithm.
Sample Solution:
Python Code:
import heapq as hq
raw_heap = [25, 44, 68, 21, 39, 23, 89]
print("Raw Heap: ", raw_heap)
hq.heapify(raw_heap)
print("\nHeapify(heap): ", raw_heap)
Sample Output:
Raw Heap: [25, 44, 68, 21, 39, 23, 89] Heapify(heap): [21, 25, 23, 44, 39, 68, 89]
Flowchart:
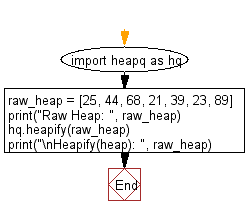
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to implement a heapsort by pushing all values onto a heap and then popping off the smallest values one at a time.
Next: Write a Python program to delete the smallest element from the given Heap and then inserts a new item.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics