Python: Find the list of words that are longer than n from a given list of words
Python List: Exercise-10 with Solution
Write a Python program to find the list of words that are longer than n from a given list of words.
Visual Presentation:
Sample Solution:
Python Code:
# Define a function called 'long_words' that takes an integer 'n' and a string 'str' as input
def long_words(n, str):
# Create an empty list 'word_len' to store words longer than 'n' characters
word_len = []
# Split the input string 'str' into a list of words using space as the delimiter
txt = str.split(" ")
# Iterate through each word 'x' in the list of words 'txt'
for x in txt:
# Check if the length of the current word 'x' is greater than 'n'
if len(x) > n:
# If the word is longer than 'n' characters, add it to the 'word_len' list
word_len.append(x)
# Return the list of words longer than 'n' characters
return word_len
# Call the 'long_words' function with an 'n' value of 3 and a string as input, and print the result
print(long_words(3, "The quick brown fox jumps over the lazy dog"))
Sample Output:
['quick', 'brown', 'jumps', 'over', 'lazy']
Explanation:
In the above exercise -
def long_words(n, str): -> Defines a function called ‘long_words’ that takes two parameters: 'n' and 'str'. 'n' is an integer that represents the minimum length of words to be returned by the function, and 'str' is a string that will be processed by the function.
word_len = [] -> Initialize an empty list called 'word_len'.
txt = str.split(" ") -> This line splits the input string 'str' into a list of words using the split() method and stores in the 'txt' variable. The argument " " passed to split() specifies that words in the string should be separated by spaces.
for x in txt: if len(x) > n: word_len.append(x)
The above lines loop through each word in the 'txt' list and check if its length is greater than n. If the length of the word is greater than n, the word is added to the word_len list using the append() method.
return word_len -> This line returns the word_len list, which contains all the words in the input string that have a length greater than n.
print(long_words(3, "The quick brown fox jumps over the lazy dog")) -> This line calls the 'long_words' function with the input parameters 3 and "The quick brown fox jumps over the lazy dog". The function returns a list of all the words in the input string that have a length greater than 3.
Finally output will be ['quick', 'brown', 'jumps', 'over', 'lazy']
Flowchart:
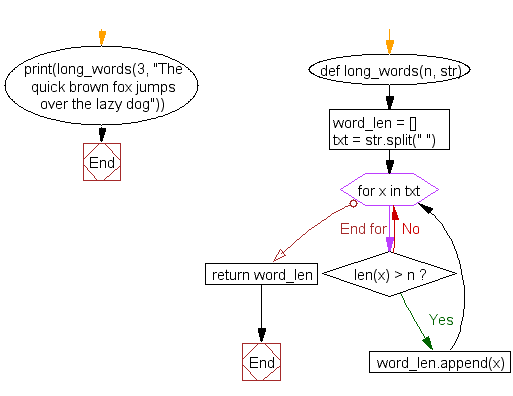
Python Code Editor:
Previous: Write a Python program to clone or copy a list.
Next: Write a Python function that takes two lists and returns True if they have at least one common member.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics