Python: Rotate a given list by specified number of items to the right or left direction
Rotate List Left or Right
Write a Python program to rotate a given list by a specified number of items in the right or left direction.
Sample Solution:
Python Code:
# Create a list 'nums1' with integers from 1 to 10
nums1 = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# Print a message indicating the original list
print("Original List:")
# Print the contents of 'nums1'
print(nums1)
# Print a message indicating that the list will be rotated to the left by 4 positions
print("\nRotate the said list in left direction by 4:")
# Perform a left rotation by slicing the list
result = nums1[3:] + nums1[:4]
# Print the rotated list
print(result)
# Print a message indicating that the list will be rotated to the left by 2 positions
print("\nRotate the said list in left direction by 2:")
# Perform a left rotation by slicing the list
result = nums1[2:] + nums1[:2]
# Print the rotated list
print(result)
# Print a message indicating that the list will be rotated to the right by 4 positions
print("\nRotate the said list in Right direction by 4:")
# Perform a right rotation by slicing the list
result = nums1[-3:] + nums1[:-4]
# Print the rotated list
print(result)
# Print a message indicating that the list will be rotated to the right by 2 positions
print("\nRotate the said list in Right direction by 2:")
# Perform a right rotation by slicing the list
result = nums1[-2:] + nums1[:-2]
# Print the rotated list
print(result)
Sample Output:
original List: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] Rotate the said list in left direction by 4: [4, 5, 6, 7, 8, 9, 10, 1, 2, 3, 4] Rotate the said list in left direction by 2: [3, 4, 5, 6, 7, 8, 9, 10, 1, 2] Rotate the said list in Right direction by 4: [8, 9, 10, 1, 2, 3, 4, 5, 6] Rotate the said list in Right direction by 2: [9, 10, 1, 2, 3, 4, 5, 6, 7, 8]
Flowchart:
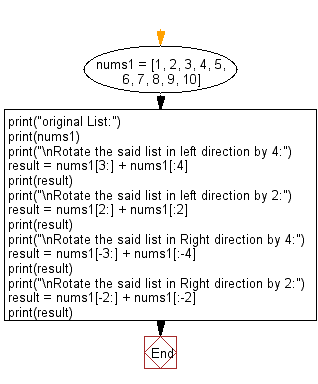
For more Practice: Solve these Related Problems:
- Write a Python program to rotate a nested list row-wise.
- Write a Python program to rotate a list while preserving even and odd positions.
- Write a Python program to rotate elements of a list based on a condition.
- Write a Python program to shift elements cyclically within a sublist.
Go to:
Previous: Write a Python program to extract a specified column from a given nested list.
Next: Write a Python program to find the item with maximum occurrences in a given list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.