Python: Check if the elements of a given list are unique or not
Check for Unique Elements in List
Write a Python program to check if the elements of a given list are unique or not.
Visual Presentation:
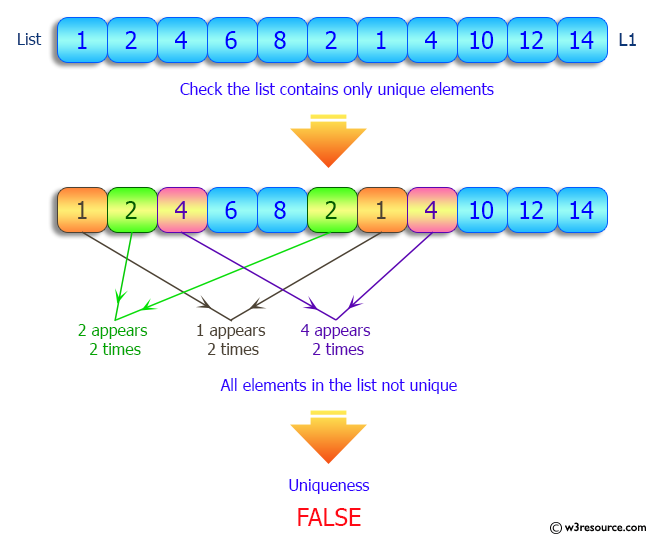
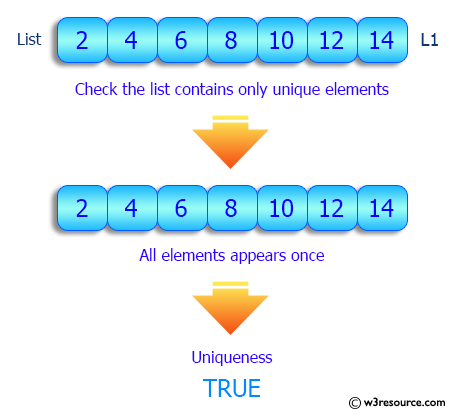
Sample Solution-1:
Python Code:
# Define a function 'all_unique' that checks if all elements in a list are unique
def all_unique(test_list):
# Check if the length of the list is greater than the length of the set of the list
if len(test_list) > len(set(test_list)):
return False
return True
# Create a list 'nums1' with duplicate elements
nums1 = [1, 2, 4, 6, 8, 2, 1, 4, 10, 12, 14, 12, 16, 17]
# Print a message indicating the original list
print("Original list:")
# Print the contents of 'nums1'
print(nums1)
# Print a message indicating the check for unique elements in the list
print("\nIs the said list contains all unique elements!")
# Call the 'all_unique' function with 'nums1' and print the result
print(all_unique(nums1))
# Create a list 'nums2' with unique elements
nums2 = [2, 4, 6, 8, 10, 12, 14]
# Print a message indicating the original list
print("\nOriginal list:")
# Print the contents of 'nums2'
print(nums2)
# Print a message indicating the check for unique elements in the list
print("\nIs the said list contains all unique elements!")
# Call the 'all_unique' function with 'nums2' and print the result
print(all_unique(nums2))
Sample Output:
Original list: [1, 2, 4, 6, 8, 2, 1, 4, 10, 12, 14, 12, 16, 17] Is the said list contains all unique elements! False Original list: [2, 4, 6, 8, 10, 12, 14] Is the said list contains all unique elements! True
Flowchart:
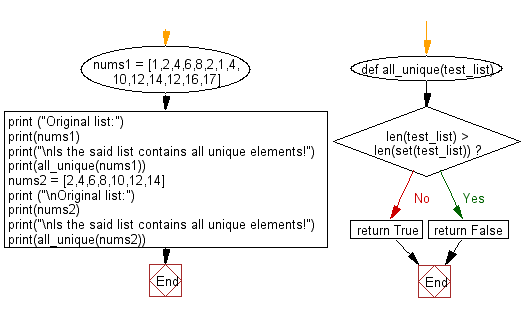
Sample Solution-2:
- Use set() on the given list to keep only unique occurrences.
- Use len() to compare the length of the unique values to the original list.
Python Code:
# Define a function 'all_unique' that checks if all elements in a list are unique
def all_unique(test_list):
# Check if the length of the list is equal to the length of the set of the list
return len(test_list) == len(set(test_list))
# Create a list 'nums1' with duplicate elements
nums1 = [1, 2, 4, 6, 8, 2, 1, 4, 10, 12, 14, 12, 16, 17]
# Print a message indicating the original list
print("Original list:")
# Print the contents of 'nums1'
print(nums1)
# Print a message indicating the check for unique elements in the list
print("\nIs the said list contains all unique elements!")
# Call the 'all_unique' function with 'nums1' and print the result
print(all_unique(nums1))
# Create a list 'nums2' with unique elements
nums2 = [2, 4, 6, 8, 10, 12, 14]
# Print a message indicating the original list
print("\nOriginal list:")
# Print the contents of 'nums2'
print(nums2)
# Print a message indicating the check for unique elements in the list
print("\nIs the said list contains all unique elements!")
# Call the 'all_unique' function with 'nums2' and print the result
print(all_unique(nums2))
Sample Output:
Original list: [1, 2, 4, 6, 8, 2, 1, 4, 10, 12, 14, 12, 16, 17] Is the said list contains all unique elements! False Original list: [2, 4, 6, 8, 10, 12, 14] Is the said list contains all unique elements! True
Flowchart:
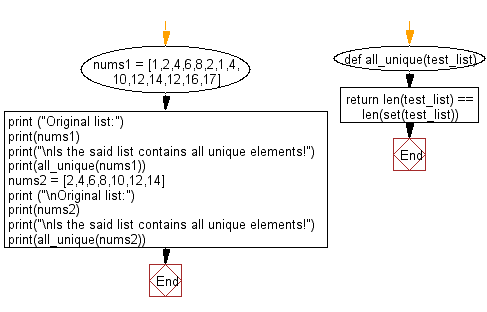
For more Practice: Solve these Related Problems:
- Write a Python program to count distinct elements in a list.
- Write a Python program to find duplicate elements in a list.
- Write a Python program to remove duplicate elements while preserving order.
- Write a Python program to check if a list contains only unique characters.
Go to:
Previous: Write a Python program to extract the nth element from a given list of tuples.
Next: Write a Python program to sort a list of lists by a given index of the inner list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.