Python: Find the difference between elements (n+1th – nth) of a given list of numeric values
Python List: Exercise - 118 with Solution
Difference Between Elements (n+1th - nth)
Write a Python program to find the difference between elements (n+1th – nth) of a given list of numeric values.
Visual Presentation:
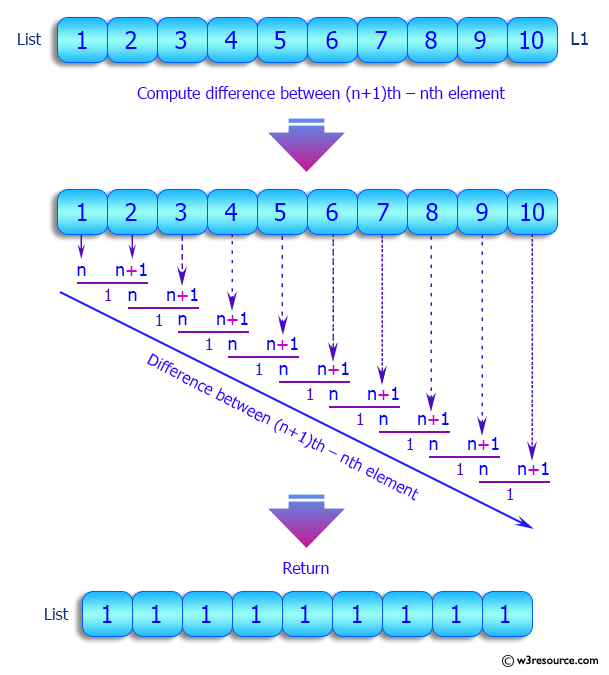
Sample Solution:
Python Code:
# Define a function 'elements_difference' that calculates the differences between adjacent elements in a list
def elements_difference(nums):
# Use a list comprehension with zip to calculate differences (n+1th – nth)
result = [j - i for i, j in zip(nums[:-1], nums[1:])]
return result
# Create two lists 'nums1' and 'nums2'
nums1 = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
nums2 = [2, 4, 6, 8]
# Print a message indicating the original list
print("Original list:")
# Print the contents of 'nums1'
print(nums1)
# Print a message indicating the operation to calculate differences
print("\nDifference between elements (n+1th – nth) of the said list:")
# Call the 'elements_difference' function with 'nums1' and print the result
print(elements_difference(nums1))
# Print a message indicating the original list
print("\nOriginal list:")
# Print the contents of 'nums2'
print(nums2)
# Print a message indicating the operation to calculate differences
print("\nDifference between elements (n+1th – nth) of the said list:")
# Call the 'elements_difference' function with 'nums2' and print the result
print(elements_difference(nums2))
Sample Output:
Original list: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] Dfference between elements (n+1th – nth) of the said list : [1, 1, 1, 1, 1, 1, 1, 1, 1] Original list: [2, 4, 6, 8] Dfference between elements (n+1th – nth) of the said list : [2, 2, 2]
Flowchart:
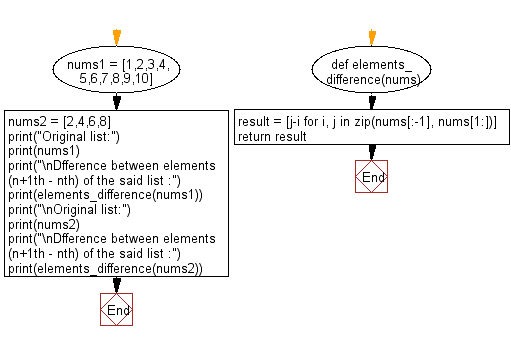
Python Code Editor:
Previous: Write a Python program to remove all elements from a given list present in another list.
Next: Write a Python program to check if a substring presents in a given list of string values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list/python-data-type-list-exercise-118.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics