Python: Reverse strings in a given list of string values
Reverse Strings in List
Write a Python program to reverse strings in a given list of string values.
Sample Solution:
Python Code:
# Define a function 'reverse_strings_list' that reverses the strings in a list
def reverse_strings_list(string_list):
# Use list comprehension to reverse each string in 'string_list'
result = [x[::-1] for x in string_list]
# Return the 'result' list with reversed strings
return result
# Create a list 'colors_list' containing string values
colors_list = ["Red", "Green", "Blue", "White", "Black"]
# Print a message indicating the original list
print("\nOriginal list:")
# Print the contents of 'colors_list'
print(colors_list)
# Print a message indicating the operation to reverse the strings in the list
print("\nReverse strings of the said given list:")
# Call the 'reverse_strings_list' function with 'colors_list' and print the result
print(reverse_strings_list(colors_list))
Sample Output:
Original lists: ['Red', 'Green', 'Blue', 'White', 'Black'] Reverse strings of the said given list: ['deR', 'neerG', 'eulB', 'etihW', 'kcalB']
Flowchart:
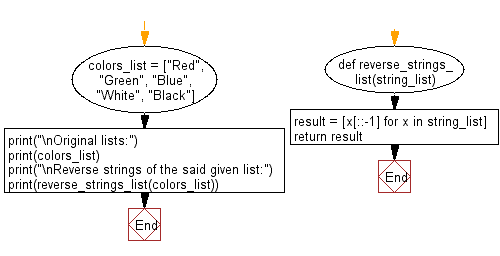
For more Practice: Solve these Related Problems:
- Write a Python program to reverse each word in a string.
- Write a Python program to check if words in a list are palindromes.
- Write a Python program to sort a list of strings in reverse order.
- Write a Python program to join reversed strings into a single sentence.
Go to:
Previous: Write a Python program to find common element(s) in a given nested lists.
Next: Write a Python program to find the maximum and minimum product from the pairs of tuple within a given list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.