Python: Find the maximum and minimum product from the pairs of tuple within a given list
Python List: Exercise - 124 with Solution
Max and Min Product of Tuple Pairs
Write a Python program to find the maximum and minimum product of pairs of tuples within a given list.
Sample Solution:
Python Code:
# Define a function 'tuple_max_val' that finds the maximum and minimum product of pairs in a list of tuples
def tuple_max_val(nums):
# Calculate the maximum product using list comprehension with abs
result_max = max([abs(x * y) for x, y in nums])
# Calculate the minimum product using list comprehension with abs
result_min = min([abs(x * y) for x, y in nums])
# Return the maximum and minimum product as a tuple
return result_max, result_min
# Create a list 'nums' containing tuples of integers
nums = [(2, 7), (2, 6), (1, 8), (4, 9)]
# Print a message indicating the original list of tuples
print("The original list, tuple:")
# Print the contents of 'nums'
print(nums)
# Print a message indicating the operation to find the maximum and minimum product
print("\nMaximum and minimum product from the pairs of the said tuple of list:")
# Call the 'tuple_max_val' function with 'nums' and print the result
print(tuple_max_val(nums))
Sample Output:
The original list, tuple : [(2, 7), (2, 6), (1, 8), (4, 9)] Maximum and minimum product from the pairs of the said tuple of list: (36, 8)
Flowchart:
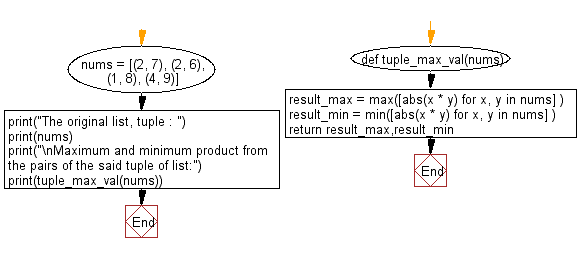
Python Code Editor:
Previous: Write a Python program to reverse strings in a given list of string values.
Next: Write a Python program to calculate the product of the unique numbers of a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list/python-data-type-list-exercise-124.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics