Python: Generate a 3D array
Generate 3D Array
Write a Python program to generate a 3*4*6 3D array whose each element is *.
Visual Presentation:
Sample Solution:
Python Code:
# Create a 3D array using list comprehensions, with 3 rows, 4 columns, and each element initialized as '*'
array = [[['*' for col in range(6)] for col in range(4)] for row in range(3)]
# Print the resulting 3D array
print(array)
Sample Output:
[[['*', '*', '*', '*', '*', '*'], ['*', '*', '*', '*', '*', '*'], ['*', '*', '*', '*', '*', '*'], ['*', '*', ' *', '*', '*', '*']], [['*', '*', '*', '*', '*', '*'], ['*', '*', '*', '*', '*', '*'], ['*', '*', '*', '*', '*' , '*'], ['*', '*', '*', '*', '*', '*']], [['*', '*', '*', '*', '*', '*'], ['*', '*', '*', '*', '*', '*'], ['*' , '*', '*', '*', '*', '*'], ['*', '*', '*', '*', '*', '*']]]
Explanation:
array = [[ ['*' for col in range(6)] for col in range(4)] for row in range(3)]
In the above code -
- The outermost range(3) loop creates 3 outermost lists, which represent the first dimension of the 3D array.
- The second range(4) loop creates 4 sub-lists for each outermost list, representing the second dimension.
- The innermost range(6) loop creates 6 innermost lists for each sub-list, representing the third dimension.
- The ' * ' character is assigned to every element of the innermost list using the list comprehension.
So, the resulting array is a 3D array of size 3x4x6, with every element initialized to the character '*'.
Finally print() function prints the said array.
Flowchart:
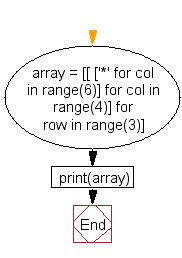
For more Practice: Solve these Related Problems:
- Write a Python program to generate a 3D array where each element is its coordinate sum.
- Write a Python program to create a 3D array where each element is a random integer between 1 and 100.
- Write a Python program to create a 3D array where each layer contains increasing numbers.
- Write a Python program to create a 3D array with alternating 0s and 1s in each row.
Go to:
Previous: Write a Python program to print a specified list after removing the 0th, 4th and 5th elements.
Next: Write a Python program to print the numbers of a specified list after removing even numbers from it.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.