Python: Print the numbers of a specified list after removing even numbers from it
Python List: Exercise - 14 with Solution
Write a Python program to print the numbers of a specified list after removing even numbers from it.
Calculating a Even Numbers:
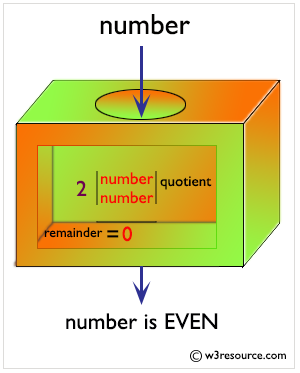
Sample Solution:
Python Code:
# Create a list 'num' containing several integer values
num = [7, 8, 120, 25, 44, 20, 27]
# Use a list comprehension to create a new list 'num' that includes only the elements from the original list
# where the element is not divisible by 2 (i.e., not even)
num = [x for x in num if x % 2 != 0]
# Print the modified 'num' list, which contains only odd values
print(num)
Sample Output:
[7, 25, 27]
Explanation:
In the above exercise -
num = [7,8, 120, 25, 44, 20, 27] -> This initializes a list named ‘num’ with the values 7, 8, 120, 25, 44, 20, 27.
num = [x for x in num if x%2!=0]
In the above code -
- This creates a new list called num by iterating over each element in the original num list using a list comprehension.
- If an element is odd (i.e., its remainder when divided by 2 is not equal to zero), it is included in the new list.
- If an element is even (i.e., its remainder when divided by 2 is equal to zero), it is excluded from the new list.
So the final output of this code will be a new list containing only the odd numbers from the original list, i.e., [7, 25, 27].
Flowchart:
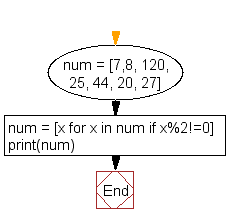
Even Numbers between 1 to 100:
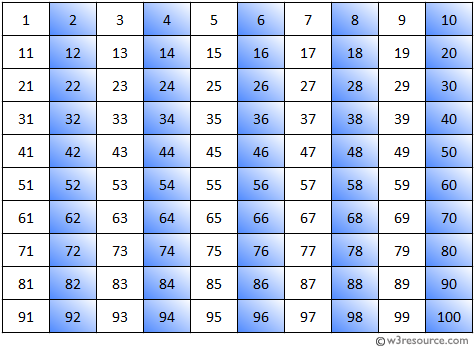
Python Code Editor:
Previous: Write a Python program to generate a 3*4*6 3D array whose each element is *.
Next: Write a Python program to shuffle and print a specified list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics