Python: Difference between the two lists
Calculate Difference Between Lists
Write a Python program to calculate the difference between the two lists.
Visual Presentation:
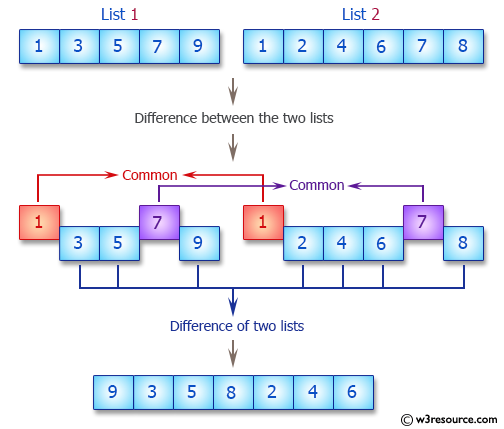
Sample Solution:
Python Code:
# Define a list 'list1' containing numbers
list1 = [1, 3, 5, 7, 9]
# Define another list 'list2' containing different numbers
list2 = [1, 2, 4, 6, 7, 8]
# Calculate the difference between 'list1' and 'list2' by converting them to sets and subtracting the sets
diff_list1_list2 = list(set(list1) - set(list2))
# Calculate the difference between 'list2' and 'list1' by converting them to sets and subtracting the sets
diff_list2_list1 = list(set(list2) - set(list1))
# Concatenate the differences 'diff_list1_list2' and 'diff_list2_list1' to obtain a list of all unique differences
total_diff = diff_list1_list2 + diff_list2_list1
# Print the total difference, which represents elements that are unique to each list
print(total_diff)
Sample Output:
[9, 3, 5, 8, 2, 4, 6]
Explanation:
In the above exercise -
- list1 = [1, 3, 5, 7, 9]: Creates a list list1 with the values [1, 3, 5, 7, 9].
- list2=[1, 2, 4, 6, 7, 8]: Creates a list list2 with the values [1, 2, 4, 6, 7, 8].
- diff_list1_list2 = list(set(list1) - set(list2)): Creates a list diff_list1_list2 containing the elements that are in list1 but not in list2. This is done by converting list1 and list2 to sets and performing set difference (-) operation between them. The resulting set is then converted back to a list diff_list1_list2.
- diff_list2_list1 = list(set(list2) - set(list1)): Creates a list diff_list2_list1 containing the elements that are in list2 but not in list1. This is done by converting list2 and list1 to sets and performing set difference (-) operation between them. The resulting set is then converted back to a list diff_list2_list1.
- total_diff = diff_list1_list2 + diff_list2_list1: Combines the elements in both difference lists diff_list1_list2 and diff_list2_list1 into a single list.
- print(total_diff): Finally print() function prints the resulting list containing the differences between the two lists, which in this case would be [3, 5, 9, 2, 4, 6, 8].
Flowchart:
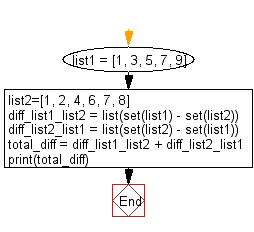
For more Practice: Solve these Related Problems:
- Write a Python program to find the intersection of two lists.
- Write a Python program to find the symmetric difference between two lists.
- Write a Python program to find the difference between two lists without using set operations.
- Write a Python program to calculate the difference between multiple lists.
Go to:
Previous: Write a Python program to generate all permutations of a list in Python.
Next: Write a Python program access the index of a list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.