Python: Check if a given function returns True for every element in a list
Check All True for Function
Write a Python program to check if a given function returns True for every element in a list.
Use all() in combination with map() and fn to check if fn returns True for all elements in the list.
Sample Solution:
Python Code:
# Define a function 'every' that takes a list 'lst' and an optional function 'fn' as input.
# The default function 'fn' is set to an identity function.
def every(lst, fn=lambda x: x):
# Use the 'all' function to check if every element in the list 'lst' satisfies the condition specified by 'fn'.
return all(map(fn, lst))
# Call the 'every' function with different lists and conditions, and print the results.
print(every([4, 2, 3], lambda x: x > 1))
print(every([4, 2, 3], lambda x: x < 1))
print(every([4, 2, 3], lambda x: x == 1))
Sample Output:
True False False
Flowchart:
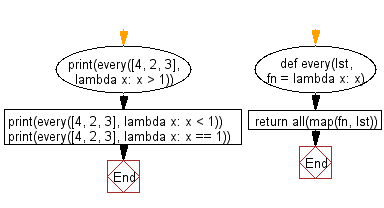
For more Practice: Solve these Related Problems:
- Write a Python program to check if a provided function returns True for every element in a list and list the indices where it fails.
- Write a Python program to verify that all elements in a list satisfy a condition defined by a lambda function and then output a boolean result.
- Write a Python program to test if a given predicate holds for all elements in a list and return a custom error message if not.
- Write a Python program to check if all elements of a list are even using a provided function, and output the result along with the count of elements checked.
Go to:
Previous: Write a Python program to get the symmetric difference between two iterables, without filtering out duplicate values.
Next: Write a Python program to initialize a list containing the numbers in the specified range where start and end are inclusive and the ratio between two terms is step. Returns an error if step equals 1.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.