Python: Find the powerset of a given iterable
Python List: Exercise - 256 with Solution
Generate Powerset of Iterable
Write a Python program to get the powerset of a given iterable.
Note: A Power Set is a set of all the subsets of a set.
- Use list() to convert the given value to a list.
- Use range() and itertools.combinations() to create a generator that returns all subsets.
- Use itertools.chain.from_iterable() and list() to consume the generator and return a list.
Sample Solution:
Python Code:
from itertools import chain, combinations
# Define a function to generate the powerset of an iterable.
def powerset(iterable):
s = list(iterable) # Convert the input iterable to a list.
return list(chain.from_iterable(combinations(s, r) for r in range(len(s) + 1)))
# Example 1
nums = [1, 2]
print("Original list elements:")
print(nums)
print("Powerset of the said list:")
print(powerset(nums))
# Example 2
nums = [1, 2, 3, 4]
print("\nOriginal list elements:")
print(nums)
print("Powerset of the said list:")
print(powerset(nums))
Sample Output:
Original list elements: [1, 2] Powerset of the said list: [(), (1,), (2,), (1, 2)] Original list elements: [1, 2, 3, 4] Powerset of the said list: [(), (1,), (2,), (3,), (4,), (1, 2), (1, 3), (1, 4), (2, 3), (2, 4), (3, 4), (1, 2, 3), (1, 2, 4), (1, 3, 4), (2, 3, 4), (1, 2, 3, 4)]
Flowchart:
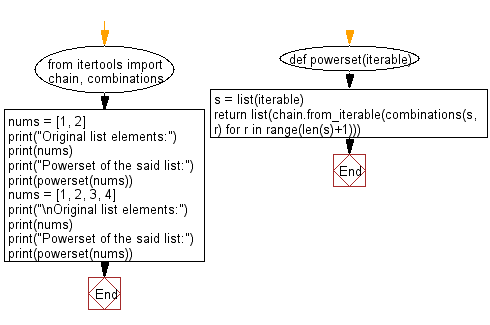
Python Code Editor:
Previous: Write a Python program to perform a deep flattens a list.
Next: Write a Python program to check if two given lists contain the same elements regardless of order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list/python-data-type-list-exercise-256.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics