Python: Generates a list of numbers in the arithmetic progression within a range
Generate Arithmetic Progression
An Arithmetic progression (AP) or arithmetic sequence is a sequence of numbers such that the difference between the consecutive terms is constant. For instance, the sequence 5, 7, 9, 11, 13, 15, . . . is an arithmetic progression with a common difference of 2.
If the initial term of an arithmetic progression is a1 and the common difference of successive members is d, then the nth term of the sequence (an) is given by:
an = a1 + (n - 1)d,
and in general
an = am + (n - m)d.
A finite portion of an arithmetic progression is called a finite arithmetic progression and sometimes just called an arithmetic progression. The sum of a finite arithmetic progression is called an arithmetic series.
Write a Python program to generate a list of numbers in the arithmetic progression starting with the given positive integer and up to the specified limit.
Use range() and list() with the appropriate start, step and end values.
Sample Solution:
Python Code:
# Define a function named 'arithmetic_progression' to generate a list of numbers in an arithmetic progression.
# It takes two parameters: 'n' is the starting number, and 'x' is the end number.
def arithmetic_progression(n, x):
# Use the 'range' function to generate a list of numbers from 'n' to 'x' (inclusive) with a step size of 'n'.
return list(range(n, x + 1, n))
# Call the 'arithmetic_progression' function to generate an arithmetic progression starting from 1 to 15 with a step of 1.
print(arithmetic_progression(1, 15))
# Call the function to generate an arithmetic progression starting from 3 to 37 with a step of 3.
print(arithmetic_progression(3, 37))
# Call the function to generate an arithmetic progression starting from 5 to 25 with a step of 5.
print(arithmetic_progression(5, 25))
Sample Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15] [3, 6, 9, 12, 15, 18, 21, 24, 27, 30, 33, 36] [5, 10, 15, 20, 25]
Flowchart:
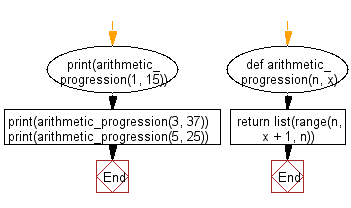
For more Practice: Solve these Related Problems:
- Write a Python program to generate an arithmetic progression given the first term, common difference, and the number of terms.
- Write a Python program to generate an arithmetic progression and calculate the sum of the series.
- Write a Python program to produce an arithmetic progression and then extract only the even terms.
- Write a Python program to generate an arithmetic progression and check if the sequence forms a palindromic pattern.
Go to:
Previous: Write a Python program to check if there are duplicate values in a given flat list.
Next: Python Dictionary Exercise Home.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.