Python: Check if there are duplicate values in a given flat list
Check Duplicates in List
Write a Python program to check if there are duplicate values in a given flat list.
Use set() on the given list to remove duplicates, compare its length with the length of the list.
Sample Solution:
Python Code:
# Define a function named 'has_duplicates' to check if a list has duplicate values.
# It takes a single parameter, 'lst', which is the list to be checked.
def has_duplicates(lst):
# Check if the length of the list is not equal to the length of the set of the list.
# If the lengths are not equal, there are duplicates in the list.
return len(lst) != len(set(lst))
# Create a list of numbers and check for duplicates.
nums = [1, 2, 3, 4, 5, 6, 7]
print("Original list:")
print(nums)
# Check if there are duplicate values in the given list using the 'has_duplicates' function.
print("Check if there are duplicate values in the said given flat list:")
print(has_duplicates(nums))
# Create another list with duplicate values and check for duplicates.
nums = [1, 2, 3, 3, 4, 5, 5, 6, 7]
print("\nOriginal list:")
print(nums)
# Check if there are duplicate values in the given list using the 'has_duplicates' function.
print("Check if there are duplicate values in the said given flat list:")
print(has_duplicates(nums))
Sample Output:
Original list: [1, 2, 3, 4, 5, 6, 7] Check if there are duplicate values in the said given flat list: False Original list: [1, 2, 3, 3, 4, 5, 5, 6, 7] Check if there are duplicate values in the said given flat list: True
Flowchart:
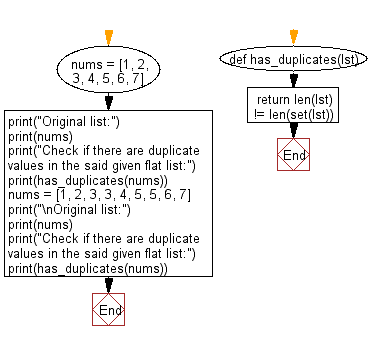
For more Practice: Solve these Related Problems:
- Write a Python program to list all duplicate elements found in a flat list.
- Write a Python program to count duplicate elements and return a dictionary mapping each duplicate to its frequency.
- Write a Python program to check for duplicates in a list without using extra data structures.
- Write a Python program to remove duplicates from a list and return both the cleaned list and the duplicates that were removed.
Go to:
Previous: Write a Python program to check if the elements of the first list are contained in the second one regardless of order.
Next: Write a Python program to generate a list of numbers in the arithmetic progression starting with the given positive integer and up to the specified limit.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.