Python: Get the frequency of the elements in a list
Count Frequency of List Elements
Write a Python program to get the frequency of elements in a list.
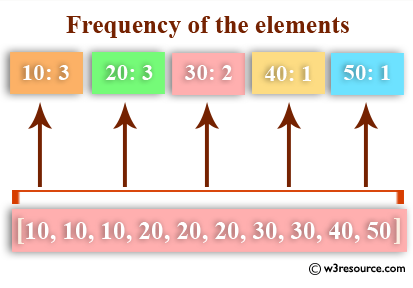
Sample Solution:
Python Code:
# Import the 'collections' module, which provides specialized container data types
import collections
# Define a list 'my_list' containing multiple numbers, including duplicates
my_list = [10, 10, 10, 10, 20, 20, 20, 20, 40, 40, 50, 50, 30]
# Print the original list 'my_list'
print("Original List : ", my_list)
# Use the 'collections.Counter' function to count the frequency of each element in 'my_list' and store it in 'ctr'
ctr = collections.Counter(my_list)
# Print the frequency of the elements in the list, as provided by the 'ctr' object
print("Frequency of the elements in the List : ", ctr)
Sample Output:
Original List : [10, 10, 10, 10, 20, 20, 20, 20, 40, 40, 50, 50, 30] Frequency of the elements in the List : Counter({10: 4, 20: 4, 40: 2, 50: 2, 30: 1})
Flowchart:
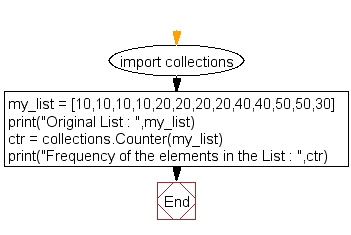
For more Practice: Solve these Related Problems:
- Write a Python program to find the most frequent element in a list.
- Write a Python program to find the least frequent element in a list.
- Write a Python program to count the frequency of list elements without using a dictionary.
- Write a Python program to count the frequency of elements and return a sorted dictionary by frequency.
Go to:
Previous: Write a Python program to get unique values from a list.
Next: Write a Python program to count the number of elements in a list within a specified range.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.