Python: Get unique values from a list
Get Unique Values from List
Write a Python program to get unique values from a list.
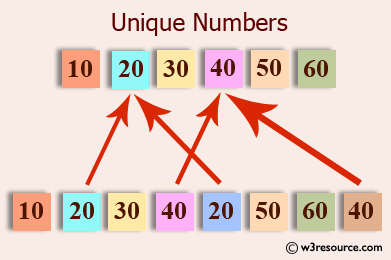
Sample Solution:
Python Code:
# Define a list 'my_list' containing multiple numbers, including duplicates
my_list = [10, 20, 30, 40, 20, 50, 60, 40]
# Print the original list 'my_list'
print("Original List : ", my_list)
# Convert the 'my_list' to a set 'my_set' to eliminate duplicates and keep unique elements
my_set = set(my_list)
# Convert the 'my_set' back to a list 'my_new_list' to obtain a list of unique numbers
my_new_list = list(my_set)
# Print the list of unique numbers stored in 'my_new_list'
print("List of unique numbers : ", my_new_list)
Sample Output:
Original List : [10, 20, 30, 40, 20, 50, 60, 40] List of unique numbers : [40, 10, 50, 20, 60, 30]
Flowchart:
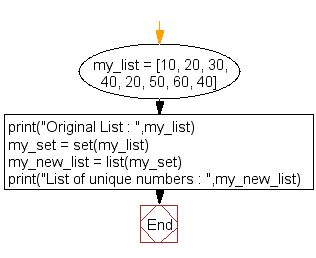
For more Practice: Solve these Related Problems:
- Write a Python program to find all unique elements in a list while maintaining order.
- Write a Python program to remove duplicate elements from a list without using sets.
- Write a Python program to get unique values from a list and count their occurrences.
- Write a Python program to get the unique elements from two lists combined.
Go to:
Previous: Write a Python program to find the second largest number in a list.
Next: Write a Python program to get the frequency of the elements in a list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.