Python: Find the items start with specific character from a given list
Find Items Starting with Specific Character
Write a Python program to find items starting with a specific character from a list.
Sample Solution:
Python Code:
# Define a function 'test' that takes a list 'lst' and a character 'char' as arguments
def test(lst, char):
# Use a list comprehension to filter elements in 'lst' that start with the specified 'char'
result = [i for i in lst if i.startswith(char)]
return result
# Define a list 'text' containing strings
text = ["abcd", "abc", "bcd", "bkie", "cder", "cdsw", "sdfsd", "dagfa", "acjd"]
# Print a message indicating the purpose of the following output
print("\nOriginal list:")
print(text)
# Define a character 'char'
char = "a"
# Print a message indicating the purpose of the following output
print("\nItems start with", char, "from the said list:")
# Call the 'test' function with 'text' and 'char' as arguments and print the result
print(test(text, char))
# Change the value of 'char' to "d"
char = "d"
# Print a message indicating the purpose of the following output
print("\nItems start with", char, "from the said list:")
# Call the 'test' function with 'text' and the updated 'char' as arguments and print the result
print(test(text, char))
# Change the value of 'char' to "w"
char = "w"
# Print a message indicating the purpose of the following output
print("\nItems start with", char, "from the said list:")
# Call the 'test' function with 'text' and the updated 'char' as arguments and print the result
print(test(text, char))
Sample Output:
Original list: ['abcd', 'abc', 'bcd', 'bkie', 'cder', 'cdsw', 'sdfsd', 'dagfa', 'acjd'] Items start with a from the said list: ['abcd', 'abc', 'acjd'] Items start with d from the said list: ['dagfa'] Items start with w from the said list: []
Flowchart:
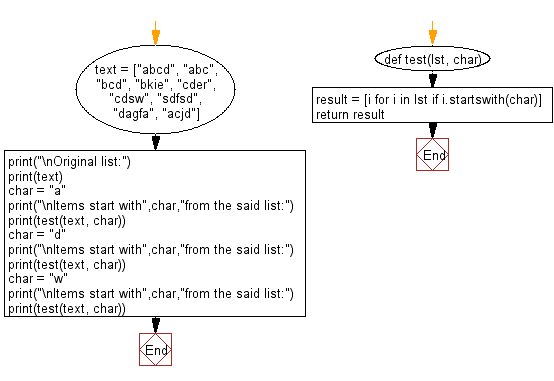
For more Practice: Solve these Related Problems:
- Write a Python program to find items ending with a specific character in a list.
- Write a Python program to find items containing a specific substring in a list.
- Write a Python program to count how many items in a list start with a specific character.
- Write a Python program to extract items starting with vowels from a list.
Go to:
Previous: Write a Python program to remove duplicates from a list of lists.
Next: Write a Python program to check if all dictionaries in a list are empty or not.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.