Python Math: Find the next previous palindrome of a specified number
Python Math: Exercise-23 with Solution
Write a Python program to find the next and previous palindromes of a specified number.
A palindromic number or numeral palindrome is a number that remains the same when its digits are reversed. Like 15951, for example, it is "symmetrical". The term palindromic is derived from palindrome, which refers to a word (such as " REDIVIDER" or even "LIVE EVIL") whose spelling is unchanged when its letters are reversed.
Sample Solution:
Python Code:
def Previous_Palindrome(num):
for x in range(num-1,0,-1):
if str(x) == str(x)[::-1]:
return x
print(Previous_Palindrome(99));
print(Previous_Palindrome(1221));
Sample Output:
88 1111
Pictorial Presentation:
Flowchart:
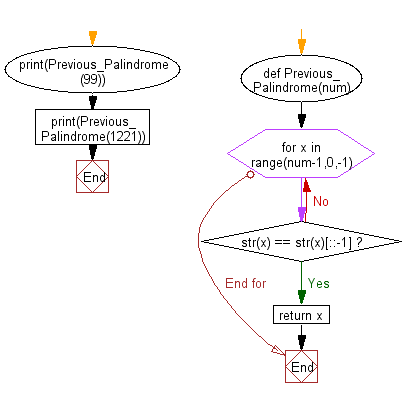
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a python program to find the next smallest palindrome of a specified number.
Next: Write a Python program to convert a float to ratio.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/math/python-math-exercise-23.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics