Python Math: Distance between two points using latitude and longitude
Python Math: Exercise-27 with Solution
Write a Python program to calculate distance between two points using latitude and longitude.
Sample Solution:
Python Code:
from math import radians, sin, cos, acos
print("Input coordinates of two points:")
slat = radians(float(input("Starting latitude: ")))
slon = radians(float(input("Ending longitude: ")))
elat = radians(float(input("Starting latitude: ")))
elon = radians(float(input("Ending longitude: ")))
dist = 6371.01 * acos(sin(slat)*sin(elat) + cos(slat)*cos(elat)*cos(slon - elon))
print("The distance is %.2fkm." % dist)
Sample Output:
Input coordinates of two points: Starting latitude: 23.5 Ending longitude: 67.5 Starting latitude: 25.5 Ending longitude: 69.5 The distance is 300.67km.
Flowchart:
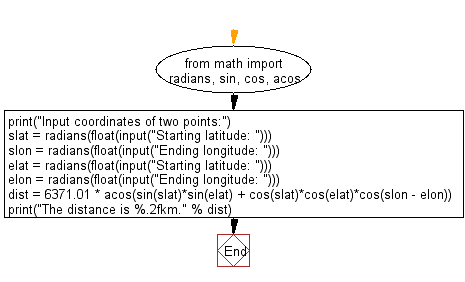
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to print number with commas as thousands separators (from right side)?
Next: Write a python program to calculate the area of a regular polygon.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/math/python-math-exercise-27.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics