Python Math: Calculate wind chill index
29. Wind Chill Index Calculator
Write a Python program to calculate the wind chill index.
Sample Solution:
Python Code:
import math
v = float(input("Input wind speed in kilometers/hour: "))
t = float(input("Input air temperature in degrees Celsius: "))
wci = 13.12 + 0.6215*t - 11.37*math.pow(v, 0.16) + 0.3965*t*math.pow(v, 0.16)
print("The wind chill index is", int(round(wci, 0)))
Sample Output:
Input wind speed in kilometers/hour: 120 Input air temperature in degrees Celsius: 35 The wind chill index is 40
Flowchart:
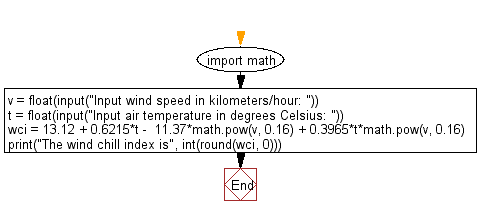
For more Practice: Solve these Related Problems:
- Write a Python program to calculate the wind chill index using the provided wind speed and air temperature, and print the result rounded to the nearest integer.
- Write a Python function that takes wind speed and temperature as input, computes the wind chill, and returns the value along with a descriptive message.
- Write a Python script to compare the wind chill index for two different sets of conditions and print which one is lower.
- Write a Python program to prompt the user for wind speed and temperature, compute the wind chill index, and then display a recommendation based on the result.
Go to:
Previous: Write a python program to calculate the area of a regular polygon.
Next: Write a Python program to find the roots of a quadratic function.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.