Python Math: Get the local and default precision
42. Local and Default Decimal Precision
Write a Python program to get the local and default precision.
Sample Solution:
Python Code:
import decimal
with decimal.localcontext() as context:
context.prec = 2
print('Local precision:', context.prec)
print('22/7 =', (decimal.Decimal('22') / 7))
print()
print('Default precision:', decimal.getcontext().prec)
print('22 /7 =', (decimal.Decimal('22') / 7))
Sample Output:
Local precision: 2 22/7 = 3.1 Default precision: 28 22 /7 = 3.142857142857142857142857143
Flowchart:
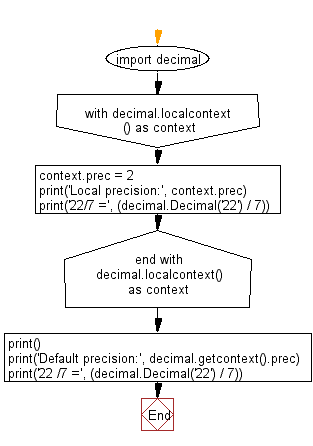
For more Practice: Solve these Related Problems:
- Write a Python program to print the local decimal context precision and the default precision from the decimal module.
- Write a Python function that changes the local Decimal context precision, prints the new context, then resets it to the default.
- Write a Python script to compare the output of a division operation under the local and default decimal precisions.
- Write a Python program to display both local and global precision settings for the decimal module and show an arithmetic operation performed under each setting.
Go to:
Previous: Write a Python program to round a specified number upwards towards infinity and down towards negative infinity of precision 4.
Next: Write a Python program to display the fraction instances of the string representation of a number.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.