Python Math: Calculate surface volume and area of a cylinder
Python Math: Exercise-5 with Solution
Write a Python program to calculate the surface volume and area of a cylinder.
Note: A cylinder is one of the most basic curvilinear geometric shapes, the surface formed by the points at a fixed distance from a given straight line, the axis of the cylinder.
Sample Solution:
Python Code:
pi=22/7
height = float(input('Height of cylinder: '))
radian = float(input('Radius of cylinder: '))
volume = pi * radian * radian * height
sur_area = ((2*pi*radian) * height) + ((pi*radian**2)*2)
print("Volume is: ", volume)
print("Surface Area is: ", sur_area)
Sample Output:
Height of cylinder: 4 Radius of cylinder: 6 Volume is: 452.57142857142856 Surface Area is: 377.1428571428571
Pictorial Presentation:
Flowchart:
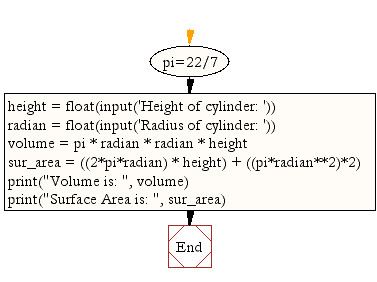
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to calculate the area of a parallelogram.
Next: Write a Python program to calculate surface volume and area of a sphere.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics