Python Math: Create an ASCII waveform
66. ASCII Waveform Generator
Write a Python program to create an ASCII waveform.
Sample Solution:-
Python Code:
from time import sleep
from math import sin, cos, radians
# increase 40 to get more wave
for n in range(1, 40):
circle_1 = 50 * (1 + sin(radians(n*10)))
circle_2 = 50 * (1 + cos(radians(n*10)))
print("#".center(int(circle_1)))
print("*".center(int(circle_2)))
sleep(0.05)
Sample Output:
# * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # * # *
Flowchart:
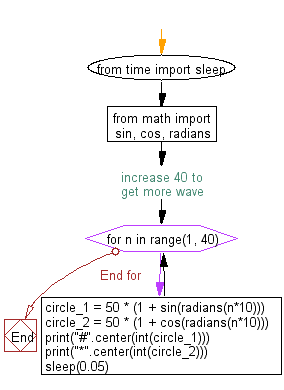
For more Practice: Solve these Related Problems:
- Write a Python program to generate an ASCII waveform from a sine function sampled at regular intervals and print the waveform.
- Write a Python function that converts an array of amplitude values into an ASCII waveform and outputs the pattern line by line.
- Write a Python script to simulate an ASCII waveform using random amplitude values and then print the resulting pattern.
- Write a Python program to create a dynamic ASCII waveform that updates every second, simulating an audio signal.
Go to:
Previous: Write a Python program to compute the value of e(2.718281827...) using infinite series.
Next: Write a Python program to create a dot string.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.