Python Math: Round up a number to specified digits
Python Math: Exercise-69 with Solution
Write a Python function to round up a number to specified digits.
Sample Solution:
Python Code:
import math
def roundup(a, digits=0):
n = 10**-digits
return round(math.ceil(a / n) * n, digits)
x = 123.01247
print("Original Number: ",x)
print(roundup(x, 0))
print(roundup(x, 1))
print(roundup(x, 2))
print(roundup(x, 3))
Sample Output:
Original Number: 123.01247 124 123.1 123.02 123.013
Flowchart:
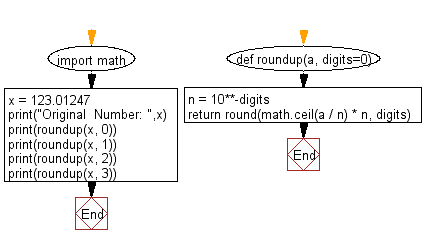
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to create a Pythagorean theorem calculator.
Next: Write a Python program for casino simulation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/math/python-math-exercise-69.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics