Python Math: Calculate arc length of an angle
7. Arc Length Calculator
Write a Python program to calculate the arc length of an angle.
Note: In a planar geometry, an angle is the figure formed by two rays, called the sides of the angle, sharing a common endpoint, called the vertex of the angle. Angles formed by two rays lie in a plane, but this plane does not have to be a Euclidean plane.
Sample Solution:
Python Code:
def arclength():
pi=22/7
diameter = float(input('Diameter of circle: '))
angle = float(input('angle measure: '))
if angle >= 360:
print("Angle is not possible")
return
arc_length = (pi*diameter) * (angle/360)
print("Arc Length is: ", arc_length)
arclength()
Sample Output:
Diameter of circle: 9 angle measure: 45 Arc Length is: 3.5357142857142856
Pictorial Presentation:
Flowchart:
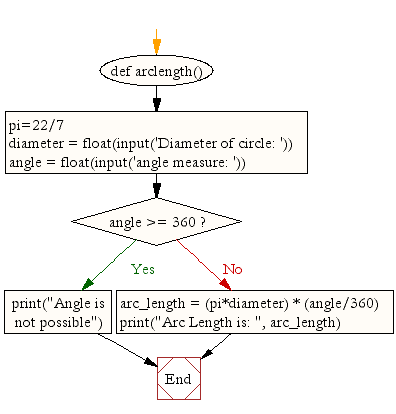
For more Practice: Solve these Related Problems:
- Write a Python program that calculates the arc length of a circle segment given the circle's diameter and an angle in degrees.
- Write a Python function that converts an angle from degrees to radians and then computes the corresponding arc length of a circle with a given diameter.
- Write a Python script to prompt the user for a circle's diameter and an angle, then output the computed arc length using the formula for arc length.
- Write a Python program to compare the arc lengths computed for two different angles in the same circle and print the difference.
Go to:
Previous: Write a Python program to calculate surface volume and area of a sphere.
Next: Write a Python program to calculate the area of a sector.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.