Python: Create a shallow copy of a given list
1. Shallow Copy of List
Write a Python program to create a shallow copy of a given list. Use copy.copy
Sample Solution:
Python Code:
import copy
nums_x = [1, [2, 3, 4]]
print("Original list: ", nums_x)
nums_y = copy.copy(nums_x)
print("\nCopy of the said list:")
print(nums_y)
print("\nChange the value of an element of the original list:")
nums_x[1][1] = 10
print(nums_x)
print("\nSecond list:")
print(nums_y)
nums = [[1], [2]]
nums_copy = copy.copy(nums)
print("\nOriginal list:")
print(nums)
print("\nCopy of the said list:")
print(nums_copy)
print("\nChange the value of an element of the original list:")
nums[0][0] = 0
print("\nFirst list:")
print(nums)
print("\nSecond list:")
print(nums_copy)
Sample Output:
Original list: [1, [2, 3, 4]] Copy of the said list: [1, [2, 3, 4]] Change the value of an element of the original list: [1, [2, 10, 4]] Second list: [1, [2, 10, 4]] Original list: [[1], [2]] Copy of the said list: [[1], [2]] Change the value of an element of the original list: First list: [[0], [2]] Second list: [[0], [2]]
Flowchart:
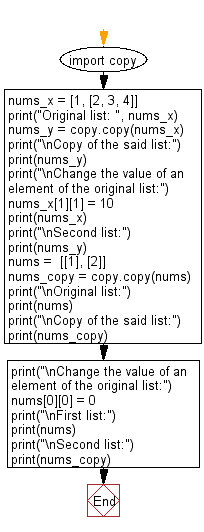
For more Practice: Solve these Related Problems:
- Write a Python program to create a shallow copy of a list containing mutable elements using copy.copy, then modify a nested element in the original list and observe the effect on the copy.
- Write a Python function that creates a shallow copy of a list using copy.copy, appends a new element to the original list, and prints both lists to show that the top-level structure of the copy remains unchanged.
- Write a Python script to compare the id() of a list and its shallow copy created with copy.copy, and then print whether they refer to the same object at the top level.
- Write a Python program to create a shallow copy of a list and then iterate over both the original and the copy, printing each element's id() to demonstrate shared references for nested objects.
Go to:
Previous: Python module Exercises Home.
Next: Write a Python program to create a deep copy of a given list.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.