Python: Create a deep copy of a given list
2. Deep Copy of List
Write a Python program to create a deep copy of a given list. Use copy.copy
Sample Solution:
Python Code:
import copy
nums_x = [1, [2, 3, 4]]
print("Original list: ", nums_x)
nums_y = copy.deepcopy(nums_x)
print("\nDeep copy of the said list:")
print(nums_y)
print("\nChange the value of an element of the original list:")
nums_x[1][1] = 10
print(nums_x)
print("\nCopy of the second list (Deep copy):")
print(nums_y)
nums = [[1, 2, 3], [4, 5, 6]]
deep_copy = copy.deepcopy(nums)
print("\nOriginal list:")
print(nums)
print("\nDeep copy of the said list:")
print(deep_copy)
print("\nChange the value of some elements of the original list:")
nums[0][2] = 55
nums[1][1] = 77
print("\nOriginal list:")
print(nums)
print("\nSecond list (Deep copy):")
print(deep_copy)
Sample Output:
Original list: [1, [2, 3, 4]] Deep copy of the said list: [1, [2, 3, 4]] Change the value of an element of the original list: [1, [2, 10, 4]] Copy of the second list (Deep copy): [1, [2, 3, 4]] Original list: [[1, 2, 3], [4, 5, 6]] Deep copy of the said list: [[1, 2, 3], [4, 5, 6]] Change the value of some elements of the original list: Original list: [[1, 2, 55], [4, 77, 6]] Second list (Deep copy): [[1, 2, 3], [4, 5, 6]]
Flowchart:
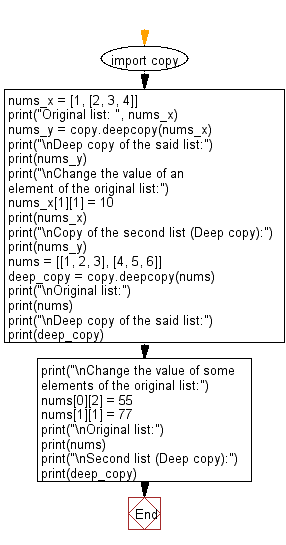
For more Practice: Solve these Related Problems:
- Write a Python program to create a deep copy of a list containing nested lists using copy.deepcopy and show that changes in the inner lists of the original do not affect the copy.
- Write a Python function that accepts a complex list (with nested dictionaries and lists), creates a deep copy using copy.deepcopy, and then prints both the original and copied lists to verify independence.
- Write a Python script to demonstrate deep copying by modifying an element within a nested list after deep copy, and then compare the nested objects' id() values between the original and the copy.
- Write a Python program to generate a deep copy of a list of mutable objects using copy.deepcopy, then perform modifications on the original list to confirm the copy remains unaltered.
Go to:
Previous: Write a Python program to create a shallow copy of a given list.
Next: Write a Python program to create a shallow copy of a given dictionary.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.