Python: Create a list of random integers and select multiple items from the said list
8. Random Sample from List
Write a Python program to create a list of random integers and randomly select multiple items from the said list.
Use random.sample
Sample Solution:
Python Code:
import random
print("Create a list of random integers:")
population = range(0, 100)
nums_list = random.sample(population, 10)
print(nums_list)
no_elements = 4
print("\nRandomly select",no_elements,"multiple items from the said list:")
result_elements = random.sample(nums_list, no_elements)
print(result_elements)
no_elements = 8
print("\nRandomly select",no_elements,"multiple items from the said list:")
result_elements = random.sample(nums_list, no_elements)
print(result_elements)
Sample Output:
Create a list of random integers: [46, 4, 45, 50, 43, 85, 74, 42, 99, 7] Randomly select 4 multiple items from the said list: [99, 45, 85, 50] Randomly select 8 multiple items from the said list: [46, 43, 74, 4, 45, 85, 99, 50]
Flowchart:
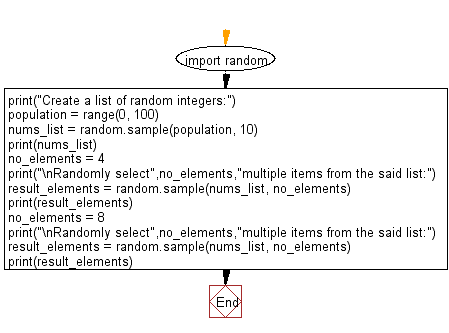
For more Practice: Solve these Related Problems:
- Write a Python program to create a list of 20 random integers and then select 5 unique items from it using random.sample().
- Write a Python script to generate a list of random elements from a given list of words and print a sample of 3 items.
- Write a Python function that takes a list and a sample size, then uses random.sample() to return a new list of randomly selected unique items.
- Write a Python program to generate a list of random integers, then randomly select and print half of them using random.sample().
Go to:
Previous: Write a Python program to generate a float between 0 and 1, inclusive and generate a random float within a specific range.
Next: Write a Python program to set a random seed and get a random number between 0 and 1.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.