Python: Set a random seed and get a random number between 0 and 1
9. Set Seed and Random Float
Write a Python program to set a random seed and get a random number between 0 and 1.
Sample Solution:
Python Code:
import random
print("Set a random seed and get a random number between 0 and 1:")
random.seed(0)
new_random_value = random.random()
print(new_random_value)
random.seed(1)
new_random_value = random.random()
print(new_random_value)
random.seed(2)
new_random_value = random.random()
print(new_random_value)
Sample Output:
Set a random seed and get a random number between 0 and 1: 0.8444218515250481 0.13436424411240122 0.9560342718892494
Flowchart:
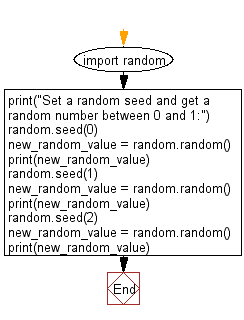
For more Practice: Solve these Related Problems:
- Write a Python program to set a specific random seed and then generate a random float between 0 and 1 using random.random(), printing the result twice to ensure consistency.
- Write a Python script to seed the random number generator, produce a random float, and then reset the seed to generate the same float again, printing both values for comparison.
- Write a Python function to set a random seed, generate a random float, and return the float along with the seed used, allowing for reproducibility tests.
- Write a Python program that demonstrates the effect of different seeds on random.random() by printing the generated floats for several seed values.
Go to:
Previous: Write a Python program to create a list of random integers and randomly select multiple items from the said list.
Next: Python Operating System Service Exercises Home.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.