Python: Check if a function is a user-defined function or not
1. User-Defined Function Checker
Write a Python program to check if a function is a user-defined function or not. Use types.FunctionType, types.LambdaType()
Sample Solution:
Python Code:
import types
def func():
return 1
print(isinstance(func, types.FunctionType))
print(isinstance(func, types.LambdaType))
print(isinstance(lambda x: x, types.FunctionType))
print(isinstance(lambda x: x, types.LambdaType))
print(isinstance(max, types.FunctionType))
print(isinstance(max, types.LambdaType))
print(isinstance(abs, types.FunctionType))
print(isinstance(abs, types.LambdaType))
Sample Output:
True True True True False False False False
Flowchart:
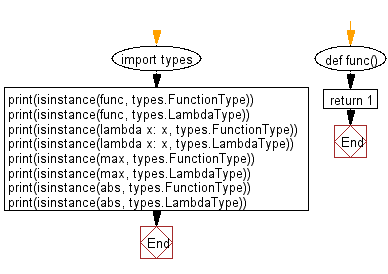
For more Practice: Solve these Related Problems:
- Write a Python program that accepts a function as input and uses types.FunctionType and types.LambdaType to determine if it is user-defined, then prints the result.
- Write a Python script to iterate over a list containing a built-in function, a lambda, and a user-defined function, and print which ones are user-defined.
- Write a Python function that distinguishes between a lambda and a regular user-defined function using types.FunctionType and types.LambdaType, outputting specific messages for each case.
- Write a Python program to define several functions (including nested functions) and use the types module to check and print whether each function is user-defined.
Go to:
Previous: Python module Exercises Home.
Next: Write a Python program to check if a given value is a method of a user-defined class.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.