Python: Check if a given value is a method of a user-defined class
2. User-Defined Method Checker
Write a Python program to check if a given value is a method of a user-defined class. Use types.MethodType()
Sample Solution:
Python Code:
import types
class C:
def x():
return 1
def y():
return 1
def b():
return 2
print(isinstance(C().x, types.MethodType))
print(isinstance(C().y, types.MethodType))
print(isinstance(b, types.MethodType))
print(isinstance(max, types.MethodType))
print(isinstance(abs, types.MethodType))
Sample Output:
True True False False False
Flowchart:
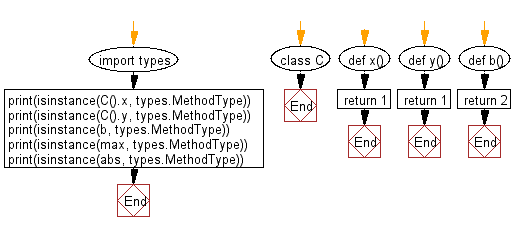
For more Practice: Solve these Related Problems:
- Write a Python program that defines a class with an instance method, retrieves that method from an object, and uses types.MethodType to confirm it is a method.
- Write a Python script to create multiple classes with various types of methods (instance, class, static) and determine using types.MethodType which ones are user-defined instance methods.
- Write a Python function that accepts any value and returns True if the value is a method of a user-defined class (using types.MethodType), otherwise False.
- Write a Python program that defines a user-defined class with a method and then checks if a dynamically assigned function (bound to an instance) is recognized as a method by types.MethodType.
Go to:
Previous: Write a Python program to check if a function is a user-defined function or not.
Next: Write a Python program to check if a given function is a generator or not.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.